设集合A、B分别用数组来表示,试写出实现集合的差运算C=A-B的算法(提示:C由所有属于A且不属于B的元素构成,算法描述须采用伪码语言书写)。
时间: 2023-05-19 19:05:02 浏览: 100
伪码如下:
1. 初始化集合C为空
2. 对于集合A中的每个元素a,判断是否属于集合B
3. 如果a不属于集合B,则将a加入集合C中
4. 返回集合C
伪代码实现:
C = []
for a in A:
if a not in B:
C.append(a)
return C
相关问题
设集合A、B分别用向量来表示,试写出实现集合的差运算C=A-B的算法 (提示:C由所有属于A且不属于B的元素构成,算法描述须采用伪码语言书写)。
伪码实现如下:
1. 创建一个新的集合C
2. 对于A中的每个元素a:
- 如果a不在B中,则将a加入C中
3. 返回C
伪代码实现示例:
set_difference(A, B):
C = new Set()
for a in A:
if a not in B:
C.add(a)
return C
注意:向量表示集合时,应将每个元素看做一个向量的维度,不需要特殊处理。
. 论述题 设集合A、B分别用向量来表示,试写出实现集合的差运算C=A-B的算法 (提示:C由所有属于A且不属于B的元素构成,算法描述须采用伪码语言书写)。
算法描述如下:
1. 初始化一个空向量C
2. 遍历向量A中的每个元素a
3. 判断a是否属于向量B,如果不属于,则将a添加到向量C中
4. 返回向量C作为集合A与B的差集
伪代码如下:
C = []
for a in A:
if a not in B:
C.append(a)
return C
阅读全文
相关推荐
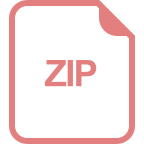
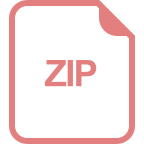
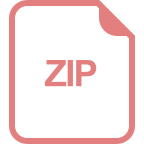













