缠论自动画笔,python代码
时间: 2023-07-23 09:43:17 浏览: 1043
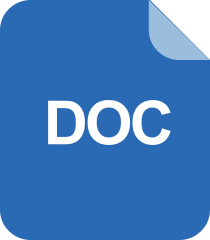
缠论画笔、线段函数通达信指标公式源码.doc

缠论是一种技术分析方法,可以用来预测股票走势。其中,自动画笔是缠论分析中的一个重要工具,可以自动识别股票走势中的笔和线段,并画出相应的趋势线。
下面是一个利用Python实现缠论自动画笔的代码示例:
```python
import numpy as np
import pandas as pd
import tushare as ts
# 获取股票数据
df = ts.get_k_data('600519', start='2020-01-01', end='2021-12-31')
# 初始化变量
bi_list = [] # 笔列表
xd_list = [] # 线段列表
bi_start = 0 # 笔起始位置
# 循环遍历K线数据
for i in range(1, len(df)):
# 判断是否为顶分型
if df.iloc[i]['high'] > df.iloc[i-1]['high'] and df.iloc[i]['high'] > df.iloc[i+1]['high']:
# 判断是否为笔
if not bi_list or df.iloc[i]['high'] > bi_list[-1]['high']:
bi_list.append({'start': bi_start, 'end': i, 'high': df.iloc[i]['high'], 'low': df.iloc[bi_start]['low'], 'type': '顶分型'})
bi_start = i
else:
bi_list[-1] = {'start': bi_start, 'end': i, 'high': df.iloc[i]['high'], 'low': df.iloc[bi_start]['low'], 'type': '顶分型'}
# 判断是否为底分型
elif df.iloc[i]['low'] < df.iloc[i-1]['low'] and df.iloc[i]['low'] < df.iloc[i+1]['low']:
# 判断是否为笔
if not bi_list or df.iloc[i]['low'] < bi_list[-1]['low']:
bi_list.append({'start': bi_start, 'end': i, 'high': df.iloc[bi_start]['high'], 'low': df.iloc[i]['low'], 'type': '底分型'})
bi_start = i
else:
bi_list[-1] = {'start': bi_start, 'end': i, 'high': df.iloc[bi_start]['high'], 'low': df.iloc[i]['low'], 'type': '底分型'}
# 判断是否为线段
if len(bi_list) >= 2 and bi_list[-2]['type'] != bi_list[-1]['type']:
xd_list.append({'start': bi_list[-2]['start'], 'end': bi_list[-1]['end'], 'high': max(bi_list[-2]['high'], bi_list[-1]['high']), 'low': min(bi_list[-2]['low'], bi_list[-1]['low'])})
# 画出线段和笔的趋势线
for xd in xd_list:
# 画出线段的趋势线
trend_line = np.polyfit([xd['start'], xd['end']], [xd['high'], xd['low']], 1)
trend_line_fn = np.poly1d(trend_line)
df.loc[xd['start']:xd['end'], 'trend_line'] = trend_line_fn(range(xd['start'], xd['end']+1))
# 画出笔的趋势线
trend_line = np.polyfit([bi_list[xd['start']]['start'], bi_list[xd['end']]['end']], [xd['high'], xd['low']], 1)
trend_line_fn = np.poly1d(trend_line)
df.loc[bi_list[xd['start']]['start']:bi_list[xd['end']]['end'], 'trend_line'] = trend_line_fn(range(bi_list[xd['start']]['start'], bi_list[xd['end']]['end']+1))
# 画出笔的实体部分
for bi in bi_list:
df.loc[bi['start']:bi['end'], 'bi'] = bi['type']
# 画出K线图和趋势线
df.plot(y=['open', 'close', 'high', 'low', 'trend_line'])
```
这段代码中,我们使用了tushare库获取股票数据,然后通过循环遍历K线数据的方式,识别出每一个笔和线段,并画出相应的趋势线和实体部分。
其中,我们使用了np.polyfit函数和np.poly1d函数来计算趋势线的斜率和截距,并通过DataFrame的loc方法来画出趋势线和实体部分。
最后,我们使用DataFrame的plot方法来画出K线图和趋势线。
阅读全文
相关推荐
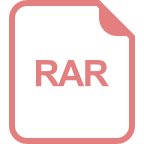
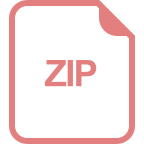
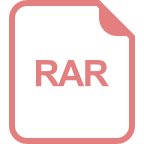




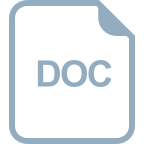
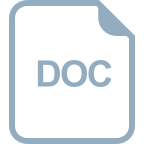


