写一个10进制转16进制c语言程序
时间: 2023-05-25 19:04:27 浏览: 79
以下是将一个10进制数转换为16进制数的C程序:
```
#include <stdio.h>
int main()
{
int num, rem, i = 0;
char hex[20];
printf("Enter a decimal number: ");
scanf("%d", &num);
while (num > 0)
{
rem = num % 16;
if (rem < 10)
hex[i] = rem + 48;
else
hex[i] = rem + 55;
i++;
num = num / 16;
}
printf("Hexadecimal equivalent: ");
for (int j = i - 1; j >= 0; j--)
printf("%c", hex[j]);
return 0;
}
```
在该程序中,我们首先接收一个十进制数并将其存储在变量`num`中。然后,我们使用一个while循环将其转换为十六进制数。在while循环中,我们计算num的余数,并将其用于查找相应的十六进制值。如果余数小于10,则我们添加48,如果余数为10或更大,则我们添加55,以获取相应的十六进制位。然后,我们将其存储在一个字符数组中,并逐个打印字符数组,以显示十六进制等效项。
相关问题
写一个十进制转十六进制的C语言代码
以下是将十进制数转换为十六进制的C语言代码:
```c
#include <stdio.h>
int main()
{
int decimal, quotient, remainder;
int i = 1, j, temp;
char hexadecimal[100];
printf("Enter a decimal number: ");
scanf("%d", &decimal);
quotient = decimal;
while (quotient != 0)
{
temp = quotient % 16;
if (temp < 10)
temp = temp + 48;
else
temp = temp + 55;
hexadecimal[i++] = temp;
quotient = quotient / 16;
}
printf("Hexadecimal equivalent is: ");
for (j = i - 1; j > 0; j--)
printf("%c", hexadecimal[j]);
return 0;
}
```
这个程序的基本思路是:
1. 首先,从用户接收一个十进制数。
2. 然后,将该数字除以16,得到商和余数。
3. 如果余数小于10,则将其转换为字符并将其添加到一个数组中。
4. 如果余数大于等于10,则将其转换为相应的字母(A、B、C、D、E或F)并将其添加到数组中。
5. 重复第2步,直到商为0。
6. 最后,从数组的末尾开始,将所有字符打印出来,以获得十六进制等效项。
使用C语言来写一个 10进制转16进制的程序
在C语言中,你可以通过内置的`itoa()`函数或者自定义转换函数来实现十进制数转换为十六进制。这里提供一个简单的示例,使用数组和字符来手动完成转换:
```c
#include <stdio.h>
#include <string.h>
// 十进制转十六进制的辅助函数
char* decimal_to_hexadecimal(int num) {
char hex[17]; // 包含空格和结束符
int i = 0;
if (num == 0) {
hex[i++] = '0';
} else {
while (num > 0) {
hex[i++] = num % 16 + '0'; // 0-9
if (hex[i - 1] > '9') { // 如果大于9,转换为A-F
hex[i - 1] += 55;
}
num /= 16;
}
if (hex[i - 1] > '9') { // 如果最高位是A-F之一,需要加前导0
hex[--i] = '0';
}
}
hex[i] = '\0'; // 添加字符串结束符
return hex;
}
int main() {
int decimal_num = 12345;
char* hex_num = decimal_to_hexadecimal(decimal_num);
printf("Decimal number: %d\n", decimal_num);
printf("Hexadecimal number: %s\n", hex_num);
free(hex_num); // 防止内存泄漏,记得释放动态分配的内存
return 0;
}
```
这个程序首先定义了一个辅助函数`decimal_to_hexadecimal`,它将输入的十进制整数转化为十六进制字符串,并返回该字符串。在`main`函数中,我们测试了这个函数。
阅读全文
相关推荐
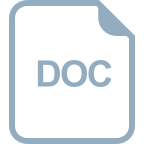
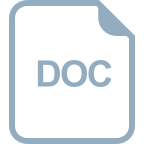











