创建多个线程并且将不同的线程绑到不同的cpu上 c语言
时间: 2024-03-13 19:46:16 浏览: 93
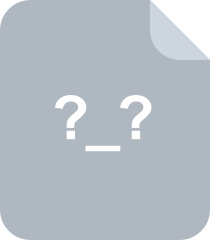
创建多线程
可以使用pthread库来创建多个线程,并使用pthread库提供的函数将线程绑定到不同的CPU上。
以下是一个示例代码,其中创建了两个线程,并将它们分别绑定到不同的CPU核心上:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define THREAD_COUNT 2
void* thread_func(void* arg) {
int tid = *(int*)arg;
printf("Thread %d started\n", tid);
// your code here
printf("Thread %d finished\n", tid);
return NULL;
}
int main() {
pthread_t threads[THREAD_COUNT];
int tids[THREAD_COUNT];
cpu_set_t cpus[THREAD_COUNT];
// set CPU affinity
CPU_ZERO(&cpus[0]);
CPU_SET(0, &cpus[0]);
CPU_ZERO(&cpus[1]);
CPU_SET(1, &cpus[1]);
// create and start threads
for (int i = 0; i < THREAD_COUNT; i++) {
tids[i] = i;
pthread_create(&threads[i], NULL, thread_func, &tids[i]);
pthread_setaffinity_np(threads[i], sizeof(cpu_set_t), &cpus[i]);
}
// wait for threads to finish
for (int i = 0; i < THREAD_COUNT; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
```
在上面的代码中,创建了两个线程,并将它们分别绑定到CPU核心0和1上。需要注意的是,在使用`pthread_setaffinity_np`函数将线程绑定到CPU上时,需要使用`pthread_t`线程ID作为第一个参数。
阅读全文
相关推荐
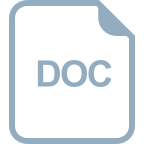
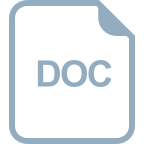
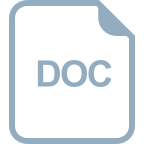
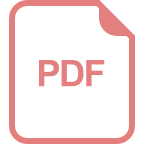
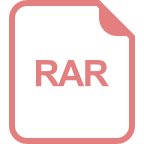
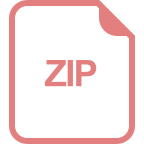
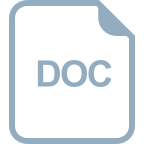
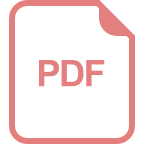
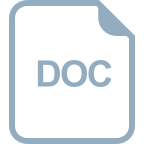
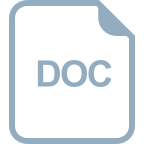
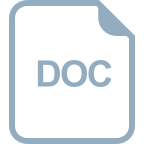
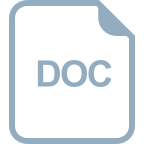
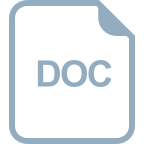
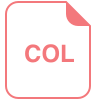
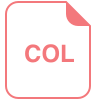
