axios如何获取vue-router的path
时间: 2023-04-04 09:03:05 浏览: 115
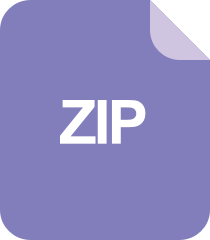
h5-my-like:vue + vue-router + vuex + axios + mock + vant + openlayers + echarts
可以通过在组件中使用this.$route.path来获取当前路由的path,然后在axios请求中使用该值作为请求的url。例如:
```
import axios from 'axios'
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter)
const router = new VueRouter({
routes: [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/about',
name: 'About',
component: About
}
]
})
axios.get(this.$route.path)
.then(response => {
console.log(response)
})
.catch(error => {
console.log(error)
})
```
阅读全文
相关推荐
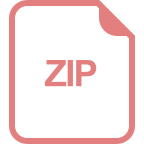
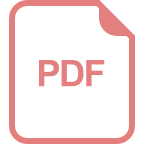
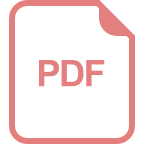
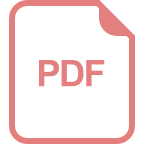
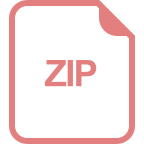
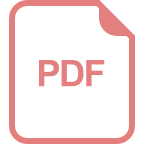
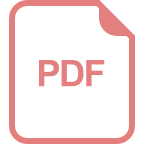
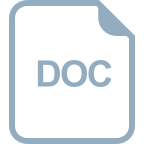
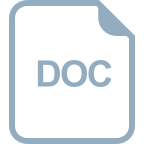




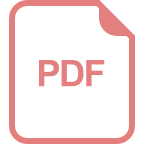
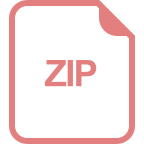
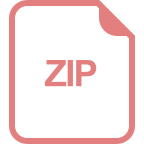
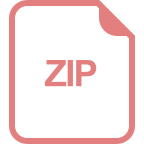