利用Python实现对两张图片按特征点重新拼接Python代码
时间: 2023-11-19 20:15:44 浏览: 90
以下是对两张图片按特征点重新拼接的Python代码示例,假设两张图片分别为img1.png和img2.png:
```python
import cv2
import numpy as np
# 读取图片
img1 = cv2.imread('img1.png')
img2 = cv2.imread('img2.png')
# 提取特征点和特征描述子
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 匹配特征点
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
# 筛选匹配点对
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# 计算单应性变换矩阵
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 变换第一张图片的位置
h, w = img1.shape[:2]
transformed_img = cv2.warpPerspective(img1, M, (w, h))
# 拼接两张图片
result = np.zeros((max(img1.shape[0], img2.shape[0]), img1.shape[1]+img2.shape[1], 3), dtype=np.uint8)
result[:img1.shape[0], :img1.shape[1]] = transformed_img
result[:img2.shape[0], img1.shape[1]:] = img2
# 显示拼接结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,以上代码仅为示例,实际应用中可能需要根据具体情况进行一些调整和优化。
阅读全文
相关推荐
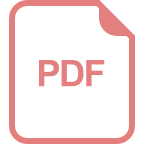

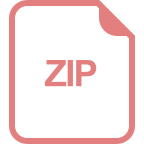
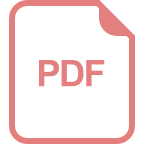
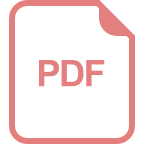
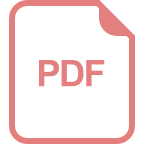








