from selenium import webdriverchrome_binary_path = "/path/to/chrome/binary"driver = webdriver.Chrome(chrome_binary=chrome_binary_path)
时间: 2023-06-20 07:04:33 浏览: 199
这是一个使用 Selenium 库打开 Chrome 浏览器的 Python 代码示例,其中 `chrome_binary_path` 是 Chrome 浏览器的可执行文件路径,可以是相对路径或绝对路径。通过 `webdriver.Chrome()` 创建一个 Chrome 浏览器对象,将其赋值给 `driver` 变量。你可以在 `driver` 上调用各种方法来模拟用户在浏览器上的操作,例如打开网页、填写表单、点击按钮等。
相关问题
from selenium import webdriver from selenium.webdriver.chrome.options import Options options=Options() options.add_argument('--headless')#设置无窗口模式 options.add_argument('--disable-gpu')#禁用gpu加速 # chrome浏览器的文件路径 path=r'C:\Program Files\Google\Chrome\Application\chrome.exe' #chrome_options.binary_location=path br=webdriver.Chrome(chrome_options=options) url='https://www.baidu.com' br.get(url) br.save_screenshot('baidu.png') 这样为什么还是会出现窗口
您好!根据您提供的代码,尽管您使用了`--headless`参数来设置无窗口模式,但是在使用Selenium时,仍然会显示浏览器窗口。这是因为在Windows操作系统中,如果Chrome浏览器已经安装在默认路径(C:\Program Files\Google\Chrome\Application\chrome.exe),那么Selenium将自动打开浏览器窗口。
为了实现完全无界面的操作,您可以尝试以下两种方法之一:
1. 指定Chrome浏览器的可执行文件路径:
您可以将`chrome_options.binary_location`的注释取消,并根据您的Chrome浏览器安装路径修改`path`变量的值。例如:
```python
path = r'your_chrome_executable_path'
options.binary_location = path
```
2. 使用Chrome WebDriver的无界面模式:
您可以下载Chrome WebDriver的无界面版本,并将其放置在Python脚本所在的目录(或者在系统PATH环境变量中)。这样,Selenium将自动使用无界面模式执行。例如,您可以从此处下载适用于您的Chrome版本的WebDriver:https://sites.google.com/a/chromium.org/chromedriver/downloads
请注意,确保您的Chrome浏览器和Chrome WebDriver的版本相匹配,以避免出现兼容性问题。
希望这些信息对您有所帮助!如果您有任何其他问题,请随时提问。
from selenium import webdriver from selenium.webdriver.chrome.service import Service from selenium.webdriver.common.by import By options = webdriver.ChromeOptions() options.add_argument('--ignore-certificate-errors') options.add_experimental_option('excludeSwitches', ['enable-automation']) options.add_argument("--disable-blink-features=AutomationControlled") options.add_argument('--disable-gpu') # 谷歌文档提到需要加上这个属性来规避bug options.add_argument('blink-settings=imagesEnabled=false') # 不加载图片, 提升速度 # options.add_argument('--headless') # 浏览器不提供可视化页面. linux下如果系统不支持可视化不加这条会启动失败 options.binary_location = './chrome-win/chrome.exe' driver_path = Service("chromedriver.exe") driver = webdriver.Chrome(service=driver_path, options=options) # 打开网站 print('正在登录!') driver.get('http://www.weather.com.cn/jiangsu/index.shtml') elements = driver.find_elements(By.XPATH, '''//*[@id="forecastID"]/dl/dd/a/b'''),from PySide2.QtWidgets import QApplication,QMainWindow from ui_main import Ui_MainWindow from PySide2.QtCore import QUrl class MainWindow(QMainWindow): def init(self): super().init() # 使用ui文件导入定义界面类 self.ui = Ui_MainWindow() # 初始化界面 self.ui.setupUi(self) # 使用界面定义的控件,也是从ui里面访问 self.ui.webview.load(QUrl('about:blank')) # 获取页面对象 page = self.ui.webview.page() # 设置页面缩放比例 page.setZoomFactor(1) self.ui.webview.load(QUrl('http://www.python3.vip/')) app = QApplication([]) mainw = MainWindow() mainw.show() app.exec_(),请合并这两段代码
你可以将这两段代码合并成一个,如下所示:
```
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from PySide2.QtWidgets import QApplication, QMainWindow
from ui_main import Ui_MainWindow
from PySide2.QtCore import QUrl
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 使用ui文件导入定义界面类
self.ui = Ui_MainWindow()
# 初始化界面
self.ui.setupUi(self)
# 使用界面定义的控件,也是从ui里面访问
self.ui.webview.load(QUrl('about:blank'))
# 获取页面对象
page = self.ui.webview.page()
# 设置页面缩放比例
page.setZoomFactor(1)
# 使用Selenium库控制Chrome浏览器
options = webdriver.ChromeOptions()
options.add_argument('--ignore-certificate-errors')
options.add_experimental_option('excludeSwitches', ['enable-automation'])
options.add_argument("--disable-blink-features=AutomationControlled")
options.add_argument('--disable-gpu') # 谷歌文档提到需要加上这个属性来规避bug
options.add_argument('blink-settings=imagesEnabled=false') # 不加载图片, 提升速度
# options.add_argument('--headless') # 浏览器不提供可视化页面. linux下如果系统不支持可视化不加这条会启动失败
options.binary_location = './chrome-win/chrome.exe'
driver_path = Service("chromedriver.exe")
driver = webdriver.Chrome(service=driver_path, options=options)
# 打开网站
print('正在登录!')
driver.get('http://www.weather.com.cn/jiangsu/index.shtml')
elements = driver.find_elements(By.XPATH, '''//*[@id="forecastID"]/dl/dd/a/b''')
if __name__ == '__main__':
app = QApplication([])
mainw = MainWindow()
mainw.show()
app.exec_()
```
注意:这段代码可能需要根据你的具体环境进行调整,比如Chrome浏览器的安装路径和版本,以及Chromedriver的文件名和路径等。
阅读全文
相关推荐
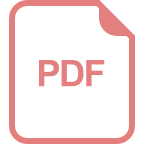
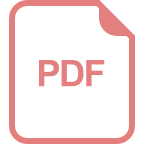
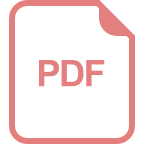









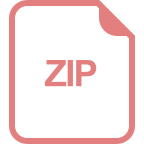
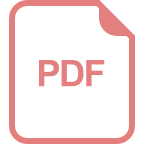
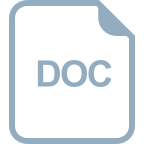
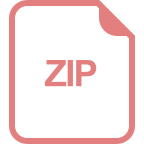
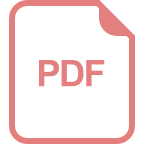