使用c语言完成双向循环链表,并实现可查找功能
时间: 2023-05-11 08:06:31 浏览: 83
好的,以下是使用 C 语言完成双向循环链表并实现可查找功能的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->prev = NULL;
newNode->next = NULL;
return newNode;
}
void insertAtEnd(Node** head, int data) {
Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
(*head)->prev = *head;
(*head)->next = *head;
return;
}
Node* lastNode = (*head)->prev;
lastNode->next = newNode;
newNode->prev = lastNode;
newNode->next = *head;
(*head)->prev = newNode;
}
Node* search(Node* head, int data) {
if (head == NULL) {
return NULL;
}
Node* curr = head;
do {
if (curr->data == data) {
return curr;
}
curr = curr->next;
} while (curr != head);
return NULL;
}
void printList(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* curr = head;
do {
printf("%d ", curr->data);
curr = curr->next;
} while (curr != head);
printf("\n");
}
int main() {
Node* head = NULL;
insertAtEnd(&head, 1);
insertAtEnd(&head, 2);
insertAtEnd(&head, 3);
insertAtEnd(&head, 4);
insertAtEnd(&head, 5);
printList(head);
Node* node = search(head, 3);
if (node != NULL) {
printf("Found node with data %d.\n", node->data);
} else {
printf("Node not found.\n");
}
return 0;
}
```
希望这个代码能够帮助你完成你的任务!
相关推荐
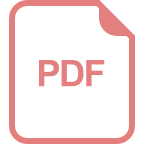
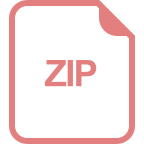
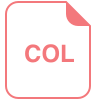
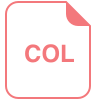
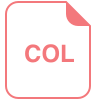
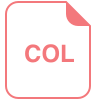
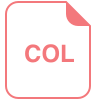









