c语言实现ECC加密算法
时间: 2023-07-13 21:39:09 浏览: 200
C语言实现ECC加密算法需要使用一些数学库来完成椭圆曲线运算,比较复杂。这里提供一个基于OpenSSL库的示例代码,用于生成密钥对和加密解密数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/evp.h>
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/bn.h>
#include <openssl/rand.h>
#define BUFSIZE 1024
int main() {
int rc = 0;
int curve_nid = NID_secp256k1;
char *message = "Hello, world!";
size_t message_len = strlen(message);
// 初始化OpenSSL库
OpenSSL_add_all_algorithms();
RAND_poll();
// 生成密钥对
EC_KEY *eckey = NULL;
eckey = EC_KEY_new_by_curve_name(curve_nid);
if (!eckey) {
printf("Error: EC_KEY_new_by_curve_name\n");
return -1;
}
if (!EC_KEY_generate_key(eckey)) {
printf("Error: EC_KEY_generate_key\n");
return -1;
}
// 显示公钥和私钥
EC_POINT *pub_key = NULL;
pub_key = EC_KEY_get0_public_key(eckey);
BIGNUM *priv_key = NULL;
priv_key = EC_KEY_get0_private_key(eckey);
char *priv_key_hex = NULL;
priv_key_hex = BN_bn2hex(priv_key);
printf("Private Key: %s\n", priv_key_hex);
char *pub_key_hex = NULL;
pub_key_hex = EC_POINT_point2hex(EC_KEY_get0_group(eckey), pub_key, POINT_CONVERSION_COMPRESSED, NULL);
printf("Public Key: %s\n", pub_key_hex);
// 加密解密数据
EVP_PKEY *privkey = EVP_PKEY_new();
if (!privkey) {
printf("Error: EVP_PKEY_new\n");
return -1;
}
if (!EVP_PKEY_set1_EC_KEY(privkey, eckey)) {
printf("Error: EVP_PKEY_set1_EC_KEY\n");
return -1;
}
EVP_PKEY *pubkey = EVP_PKEY_new();
if (!pubkey) {
printf("Error: EVP_PKEY_new\n");
return -1;
}
if (!EVP_PKEY_set1_EC_KEY(pubkey, eckey)) {
printf("Error: EVP_PKEY_set1_EC_KEY\n");
return -1;
}
EVP_CIPHER_CTX *ctx = EVP_CIPHER_CTX_new();
if (!ctx) {
printf("Error: EVP_CIPHER_CTX_new\n");
return -1;
}
unsigned char iv[BUFSIZE] = {0};
unsigned char out[BUFSIZE] = {0};
int outlen;
if (!EVP_EncryptInit_ex(ctx, EVP_aes_128_cbc(), NULL, NULL, iv)) {
printf("Error: EVP_EncryptInit_ex\n");
return -1;
}
if (!EVP_EncryptUpdate(ctx, out, &outlen, (unsigned char *)message, message_len)) {
printf("Error: EVP_EncryptUpdate\n");
return -1;
}
if (!EVP_EncryptFinal_ex(ctx, out + outlen, &outlen)) {
printf("Error: EVP_EncryptFinal_ex\n");
return -1;
}
unsigned char *encrypted_data = NULL;
size_t encrypted_data_len = outlen + message_len;
encrypted_data = (unsigned char *)malloc(encrypted_data_len);
if (!encrypted_data) {
printf("Error: malloc\n");
return -1;
}
memcpy(encrypted_data, out, outlen);
memcpy(encrypted_data + outlen, message, message_len);
unsigned char decrypted_data[BUFSIZE] = {0};
int decrypted_data_len = 0;
if (!EVP_DecryptInit_ex(ctx, EVP_aes_128_cbc(), NULL, NULL, iv)) {
printf("Error: EVP_DecryptInit_ex\n");
return -1;
}
if (!EVP_DecryptUpdate(ctx, decrypted_data, &decrypted_data_len, encrypted_data, encrypted_data_len)) {
printf("Error: EVP_DecryptUpdate\n");
return -1;
}
if (!EVP_DecryptFinal_ex(ctx, decrypted_data + decrypted_data_len, &decrypted_data_len)) {
printf("Error: EVP_DecryptFinal_ex\n");
return -1;
}
// 显示加密和解密结果
printf("Plain Text: %s\n", message);
printf("Cipher Text: ");
for (int i = 0; i < encrypted_data_len; i++) {
printf("%02x", encrypted_data[i]);
}
printf("\n");
printf("Decrypted Text: %s\n", decrypted_data);
// 释放资源
free(encrypted_data);
EC_KEY_free(eckey);
EVP_PKEY_free(privkey);
EVP_PKEY_free(pubkey);
EVP_CIPHER_CTX_free(ctx);
OPENSSL_cleanup();
return 0;
}
```
需要使用OpenSSL库来实现ECC加密算法,可以通过以下命令安装:
```bash
sudo apt-get install libssl-dev
```
编译以上代码示例:
```bash
gcc -o ecc ecc.c -lssl -lcrypto
```
注意:以上代码示例仅供参考,实际使用时需要根据具体情况进行调整。
阅读全文
相关推荐
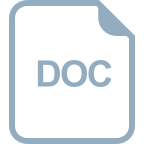
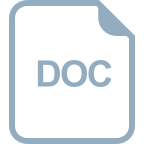
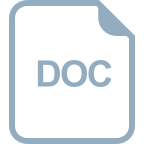
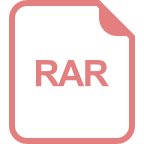
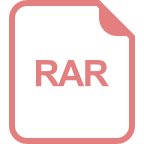
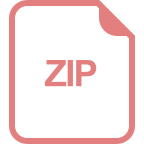





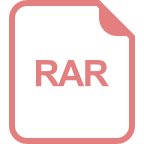
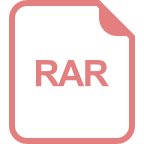
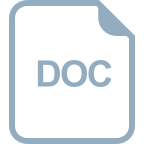
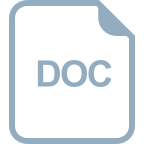



