完成图片的复制,要求1、请用户输入图片的源路径(提示用\);判断图片是否存在,如果不存在,要求用户重新输入;2、要求用户输入目的目录,判断是否是一个文件,如果不是文件,在目的路径后加上文件名;
时间: 2024-03-26 09:41:19 浏览: 24
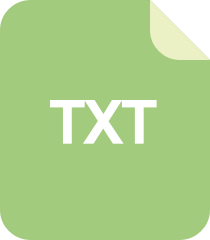
上传图片,判断上传路径是否存在如果不存在则创建,并且上传图片

好的,我理解了你的问题。以下是 Python 代码实现:
```python
import os
import shutil
# 读取源路径
while True:
src_path = input("请输入图片的源路径(用\\分隔路径):")
if not os.path.exists(src_path):
print("路径不存在,请重新输入!")
else:
break
# 读取目标路径
while True:
dst_path = input("请输入目标目录:")
if os.path.isfile(dst_path):
print("目标路径不能为文件,请重新输入!")
elif os.path.isdir(dst_path):
dst_path = os.path.join(dst_path, os.path.basename(src_path))
break
else:
break
# 复制图片
try:
shutil.copy(src_path, dst_path)
print("复制成功!")
except Exception as e:
print("复制失败:", e)
```
请注意,以上代码只能复制文件,如果需要复制文件夹,需要使用 `shutil.copytree()` 函数。
阅读全文
相关推荐
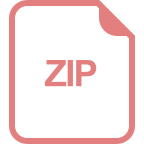


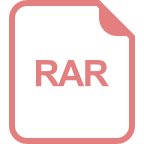
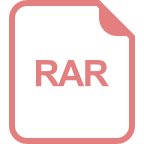
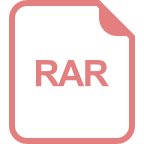
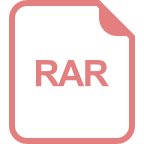
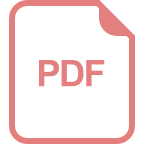
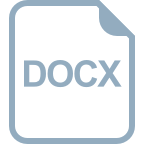
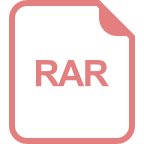
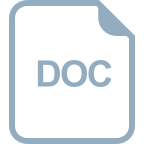