golang实现simhash算法
时间: 2023-10-25 20:08:07 浏览: 72
可以使用golang的hash/crc32包来计算simhash算法中的哈希值,具体实现可以参考以下代码:
```
package simhash
import (
"bytes"
"hash/crc32"
)
// Simhash represents a 64-bit hash value
type Simhash uint64
// NewSimhash calculates the simhash of the given data
func NewSimhash(data []byte) Simhash {
// Step 1: tokenize data into shingles
shingles := tokenize(data)
// Step 2: compute the weighted frequency counts of shingles
counts := countShingles(shingles)
// Step 3: create the simhash
var simhash Simhash
for shingle, weight := range counts {
hash := crc32.ChecksumIEEE([]byte(shingle))
for i := 0; i < 32; i++ {
if hash&(1<<uint(i)) != 0 {
simhash += Simhash(weight)
} else {
simhash -= Simhash(weight)
}
}
}
return simhash
}
// tokenize splits the given data into shingles
func tokenize(data []byte) []string {
shingles := make([]string, 0)
var buffer bytes.Buffer
for i := 0; i < len(data); i++ {
if data[i] == ' ' {
shingles = append(shingles, buffer.String())
buffer.Reset()
continue
}
buffer.WriteByte(data[i])
}
if buffer.Len() > 0 {
shingles = append(shingles, buffer.String())
}
return shingles
}
// countShingles computes the weighted frequency counts of the given shingles
func countShingles(shingles []string) map[string]int {
counts := make(map[string]int)
for _, shingle := range shingles {
counts[shingle]++
}
return counts
}
```
这段代码实现了simhash算法的三个步骤:将输入数据分割为shingles,计算shingles的加权频率,以及计算simhash值。其中,crc32包用于计算哈希值,bytes包用于处理字符串。
相关推荐
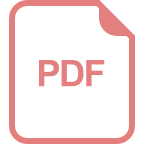
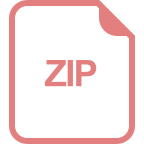
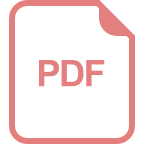














