java.io.bufferedoutputstream
时间: 2023-04-30 18:06:08 浏览: 67
java.io.BufferedOutputStream是Java标准库中的一个类,它可以将数据写入到缓冲区中,从而提高了文件写入的效率。它可以作为其他类的OutputStream的包装器,例如FileOutputStream。
相关问题
package src; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import javax.swing.JButton; import javax.swing.JFileChooser; import javax.swing.JFrame; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTextArea; public class Test3 extends JFrame { JTextArea txta=new JTextArea(10,21); JScrollPane scrp=new JScrollPane(txta); JButton btn1=new JButton("读t3_1.txt到文本框"); JButton btn2=new JButton("保存到t3_2.txt"); JPanel p=new JPanel(); public Test3() { p.add(scrp); p.add(btn1); p.add(btn2); this.setContentPane(p); this.setSize(300,270); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setLocationRelativeTo(null); this.setVisible(true); } public static void main(String[] args) { Test3 fr=new Test3(); fr.setTitle("第3题"); } }补全上述代码
可以按照以下步骤进行代码的补全:
1. 在Test3类中添加一个构造方法,然后在方法中为btn1和btn2添加监听器,用于实现读取和保存文件的功能。
2. 在读取文件的监听器中,可以使用JFileChooser组件选择文件,然后使用FileInputStream和BufferedInputStream读取文件内容,并将内容显示在文本框中。
3. 在保存文件的监听器中,可以使用JFileChooser组件选择保存文件的路径和文件名,然后使用FileOutputStream和BufferedOutputStream将文本框中的内容写入到文件中。
完整代码如下:
```
package src;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class Test3 extends JFrame {
JTextArea txta = new JTextArea(10, 21);
JScrollPane scrp = new JScrollPane(txta);
JButton btn1 = new JButton("读t3_1.txt到文本框");
JButton btn2 = new JButton("保存到t3_2.txt");
JPanel p = new JPanel();
public Test3() {
p.add(scrp);
p.add(btn1);
p.add(btn2);
this.setContentPane(p);
this.setSize(300, 270);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLocationRelativeTo(null);
this.setVisible(true);
// 为btn1添加监听器,读取文件内容
btn1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 创建文件选择器
JFileChooser chooser = new JFileChooser();
chooser.setDialogTitle("选择文件");
int result = chooser.showOpenDialog(Test3.this);
if (result == JFileChooser.APPROVE_OPTION) {
try {
// 读取文件内容
File file = chooser.getSelectedFile();
FileInputStream fis = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(fis);
byte[] buffer = new byte[(int) file.length()];
bis.read(buffer);
String text = new String(buffer);
txta.setText(text);
bis.close();
fis.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(Test3.this, "读取文件失败");
}
}
}
});
// 为btn2添加监听器,保存文件内容
btn2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 创建文件选择器
JFileChooser chooser = new JFileChooser();
chooser.setDialogTitle("保存文件");
int result = chooser.showSaveDialog(Test3.this);
if (result == JFileChooser.APPROVE_OPTION) {
try {
// 写入文件内容
File file = chooser.getSelectedFile();
FileOutputStream fos = new FileOutputStream(file);
BufferedOutputStream bos = new BufferedOutputStream(fos);
String text = txta.getText();
byte[] buffer = text.getBytes();
bos.write(buffer);
bos.close();
fos.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(Test3.this, "保存文件失败");
}
}
}
});
}
public static void main(String[] args) {
Test3 fr = new Test3();
fr.setTitle("第3题");
}
}
```
java 解压.zip
在Java中解压.zip文件可以使用java.util.zip包中的ZipInputStream类和ZipEntry来实现。下面是一个简单的示例代码:
```java
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class UnzipExample {
public static void main(String[] args) {
String zipFilePath = "path/to/your/file.zip";
String destDir = "path/to/destination/directory";
try {
File destDirectory = new File(destDir);
if (!destDirectory.exists()) {
destDirectory.mkdir();
}
FileInputStream fis = new FileInputStream(zipFilePath);
ZipInputStream zis = new ZipInputStream(fis);
ZipEntry zipEntry = zis.getNextEntry();
while (zipEntry != null) {
String entryName = zipEntry.getName();
String filePath = destDir + File.separator + entryName;
if (!zipEntry.isDirectory()) {
extractFile(zis, filePath);
} else {
File dir = new File(filePath);
dir.mkdir();
}
zis.closeEntry();
zipEntry = zis.getNextEntry();
}
zis.close();
fis.close();
System.out.println("解压完成!");
} catch (IOException e) {
e.printStackTrace();
}
}
private static void extractFile(ZipInputStream zis, String filePath) throws IOException {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(filePath));
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = zis.read(buffer)) != -1) {
bos.write(buffer, 0, bytesRead);
}
bos.close();
}
}
```
你需要将上述代码中的"path/to/your/file.zip"替换为你要解压的.zip文件的路径,将"path/to/destination/directory"替换为你要将解压后的文件保存的目标目录路径。
相关推荐
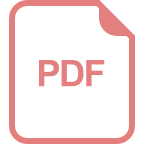
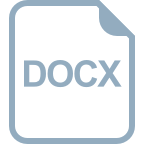
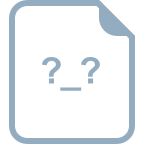
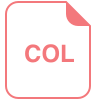
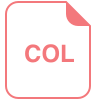
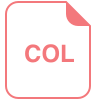
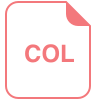
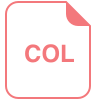







