如何构建InceptionV3模型代码
时间: 2024-05-13 07:17:49 浏览: 10
要构建InceptionV3模型,可以使用Python编写代码并使用TensorFlow框架。以下是一个简单的实现示例:
首先,导入所需的库:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, Dropout, Flatten, Dense, concatenate
from tensorflow.keras.models import Model
```
然后,定义输入张量:
```python
input_shape = (224, 224, 3)
input_tensor = Input(shape=input_shape)
```
接下来,定义Inception模块中的各个层:
```python
def conv2d_bn(x, filters, kernel_size, padding='same', strides=(1, 1)):
x = Conv2D(filters, kernel_size, strides=strides, padding=padding)(x)
x = tf.keras.layers.BatchNormalization()(x)
x = tf.keras.layers.Activation('relu')(x)
return x
def inception_module(x, filters):
branch_1 = conv2d_bn(x, filters[0], (1, 1))
branch_2 = conv2d_bn(x, filters[1], (1, 1))
branch_2 = conv2d_bn(branch_2, filters[2], (3, 3))
branch_3 = conv2d_bn(x, filters[3], (1, 1))
branch_3 = conv2d_bn(branch_3, filters[4], (5, 5))
branch_4 = MaxPooling2D((3, 3), strides=(1, 1), padding='same')(x)
branch_4 = conv2d_bn(branch_4, filters[5], (1, 1))
x = concatenate([branch_1, branch_2, branch_3, branch_4], axis=3)
return x
```
在上面的代码中,我们定义了一个辅助函数conv2d_bn,它用于完成卷积、批归一化和激活操作。然后我们定义了inception_module函数,它将输入张量作为x,并使用5个卷积核来并行处理x。最后我们通过concatenate函数将这些处理结果组合在一起。
接下来,我们可以定义整个InceptionV3模型:
```python
def inception_v3(input_shape, num_classes):
input_tensor = Input(shape=input_shape)
# Stem
x = conv2d_bn(input_tensor, 32, (3, 3), strides=(2, 2))
x = conv2d_bn(x, 32, (3, 3))
x = conv2d_bn(x, 64, (3, 3), padding='same')
x = MaxPooling2D((3, 3), strides=(2, 2))(x)
x = conv2d_bn(x, 80, (1, 1))
x = conv2d_bn(x, 192, (3, 3))
x = MaxPooling2D((3, 3), strides=(2, 2))(x)
# 3 x Inception module
x = inception_module(x, [64, 96, 128, 16, 32, 32])
x = inception_module(x, [128, 128, 192, 32, 96, 64])
x = inception_module(x, [192, 96, 208, 16, 48, 64])
# 2 x Inception module
x = inception_module(x, [160, 112, 224, 24, 64, 64])
x = inception_module(x, [128, 128, 256, 24, 64, 64])
# 2 x Inception module
x = inception_module(x, [112, 144, 288, 32, 64, 64])
x = inception_module(x, [256, 160, 320, 32, 128, 128])
x = MaxPooling2D((3, 3), strides=(2, 2))(x)
x = conv2d_bn(x, 1280, (1, 1))
x = Dropout(0.4)(x)
# Output
x = Flatten()(x)
x = Dense(num_classes, activation='softmax')(x)
model = Model(inputs=input_tensor, outputs=x, name='inception_v3')
return model
```
在这个函数中,我们首先定义了输入张量,并使用一些卷积和池化层来进行初始处理。接下来,我们使用多个inception_module函数来构建整个模型。最后,我们添加一个全局平均池化层,一个dropout层和一个全连接层来产生最终的输出。
现在我们可以使用inception_v3函数来创建一个模型:
```python
model = inception_v3(input_shape=(224, 224, 3), num_classes=1000)
```
这里我们假设我们要用InceptionV3模型对1000个类别进行分类,因此num_classes参数设置为1000。
这就是构建InceptionV3模型的基本代码。当然,为了实现更好的性能,你可能需要对模型进行调整和优化。
相关推荐














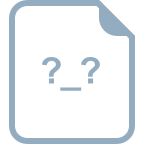