以http://192.168.3.223:8889/manage/manu/import写文件上传端口为例写一个模拟
时间: 2023-12-28 19:03:53 浏览: 106
假设我们要模拟一个文件上传的请求,使用Python的requests库可以轻松实现:
```python
import requests
url = 'http://192.168.3.223:8889/manage/manu/import'
filename = 'example.csv'
files = {'file': open(filename, 'rb')}
response = requests.post(url, files=files)
# 检查上传是否成功
if response.status_code == 200:
print("文件上传成功")
else:
print("文件上传失败")
```
这段代码中,我们首先指定上传文件的地址 `url` 和要上传的文件名 `filename`,然后使用Python内置的 `open` 函数打开文件,并通过 `files` 参数将文件传递给 `requests.post` 函数。最后,我们可以检查服务器返回的状态码来判断上传是否成功。
相关问题
爬取https://top.zol.com.cn/compositor/16/notebook.html和https://top.zol.com.cn/compositor/16/manu_attention.html 需要爬取名称,品牌,价格,评分
好的,以下是Python代码,需要安装requests、beautifulsoup4和pandas库:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 爬取第一个网页
url1 = "https://top.zol.com.cn/compositor/16/notebook.html"
resp1 = requests.get(url1)
soup1 = BeautifulSoup(resp1.text, "html.parser")
items1 = soup1.find_all("div", class_="list-item")
# 爬取第二个网页
url2 = "https://top.zol.com.cn/compositor/16/manu_attention.html"
resp2 = requests.get(url2)
soup2 = BeautifulSoup(resp2.text, "html.parser")
items2 = soup2.find_all("div", class_="list-item")
# 保存数据到Excel文件
data = []
for item in items1 + items2:
name = item.find("h3").text.strip()
brand = item.find("span", class_="item-brand").text.strip()
price = item.find("b").text.strip()
score = item.find("em").text.strip()
data.append([name, brand, price, score])
df = pd.DataFrame(data, columns=["名称", "品牌", "价格", "评分"])
df.to_excel("data.xlsx", index=False)
print("数据已保存到data.xlsx文件")
```
以上代码会将爬取的数据保存到当前目录下的data.xlsx文件中,你可以根据自己的需求修改保存的文件名和路径。
https://manu44.magtech.com.cn/Jwk_infotech_wk3/article/2023/2096-3467/2096-3467-7-4-145.shtml 获取网页的文本内容
您可以使用Python中的requests和BeautifulSoup库来获取网页的文本内容。以下是一个示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://manu44.magtech.com.cn/Jwk_infotech_wk3/article/2023/2096-3467/2096-3467-7-4-145.shtml"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text()
print(text)
```
这里我们首先使用requests库获取网页的HTML代码,然后使用BeautifulSoup库解析HTML代码,并使用get_text()方法获取网页的文本内容。最后打印出文本内容即可。
阅读全文
相关推荐
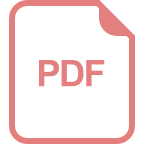
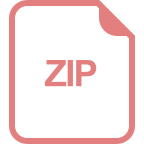
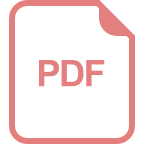
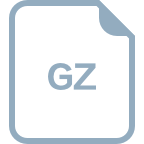
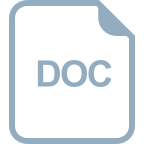
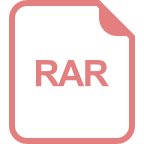
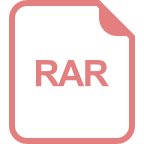
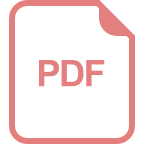
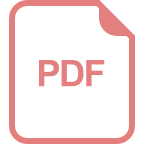
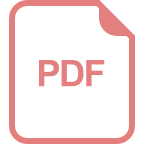
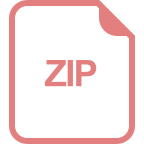
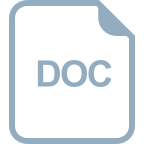
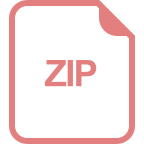
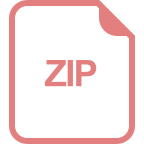
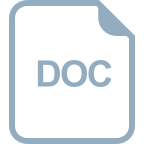
