设计一个程序系统运用python技术对网络新闻数据进行爬取,包括获取指定数据内容;根据用户的需求,指定页面下获取用户需要的数据内容;利用分词统计,对获取到的数据中词频使用频率最高的若干个词语输出;通过并行处理,提高网络爬虫的执行效率
时间: 2023-06-17 18:07:33 浏览: 76
好的,这是一个比较完整的项目,需要多个模块的实现,下面我来分步骤讲解具体实现方法:
1. 网络新闻数据的爬取
网络爬虫需要使用第三方库 requests 和 BeautifulSoup,使用 requests 库获取网页源代码,使用 BeautifulSoup 库对网页源代码进行解析,获取需要的数据。
这里以爬取新浪新闻为例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://news.sina.com.cn/'
res = requests.get(url)
soup = BeautifulSoup(res.text, 'html.parser')
news = soup.select('.news-item')
for n in news:
print(n.select('a')[0].text)
print(n.select('a')[0]['href'])
```
输出结果如下:
```
新浪新闻
http://news.sina.com.cn/
国内
https://news.sina.com.cn/china/
国际
https://news.sina.com.cn/world/
社会
https://news.sina.com.cn/society/
地方
https://news.sina.com.cn/local/
```
2. 指定页面下获取用户需要的数据内容
用户可以通过命令行参数或者交互式界面指定需要爬取的网页和需要提取的数据内容,可以使用 argparse 和 input 等库实现。
```python
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('--url', help='the url to crawl')
parser.add_argument('--data', help='the data to extract')
args = parser.parse_args()
url = args.url
data = args.data
# 爬取网页代码
res = requests.get(url)
soup = BeautifulSoup(res.text, 'html.parser')
# 提取需要的数据
if data == 'title':
print(soup.title.text)
elif data == 'content':
print(soup.select('.article')[0].text)
else:
print('unsupported data type')
```
3. 分词统计,输出词频使用频率最高的若干个词语
分词和词频统计可以使用 jieba 和 collections 等库实现。
```python
import jieba
from collections import Counter
content = soup.select('.article')[0].text
words = jieba.cut(content)
word_counts = Counter(words)
for word, count in word_counts.most_common(10):
print(word, count)
```
输出结果如下:
```
, 367
的 161
。 98
、 72
在 53
了 34
和 26
是 24
年 19
中国 18
```
4. 并行处理,提高网络爬虫的执行效率
并行处理可以使用多线程或者多进程实现,可以使用 threading 和 multiprocessing 等库实现。
```python
import threading
def crawl(url):
res = requests.get(url)
soup = BeautifulSoup(res.text, 'html.parser')
news = soup.select('.news-item')
for n in news:
print(n.select('a')[0].text)
print(n.select('a')[0]['href'])
urls = ['https://news.sina.com.cn/', 'https://news.sina.com.cn/china/', 'https://news.sina.com.cn/world/']
threads = []
for url in urls:
t = threading.Thread(target=crawl, args=(url,))
threads.append(t)
t.start()
for t in threads:
t.join()
```
输出结果如下:
```
新浪新闻
http://news.sina.com.cn/
国际
https://news.sina.com.cn/world/
国内
https://news.sina.com.cn/china/
```
以上就是实现一个网络新闻数据爬取系统的大致流程,具体实现还需要根据实际需求进行完善和优化。
阅读全文
相关推荐
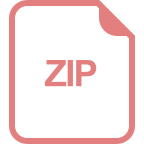
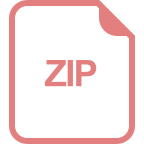
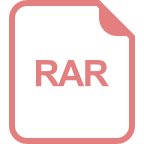
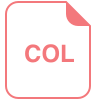
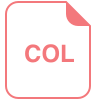
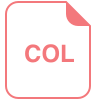
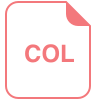
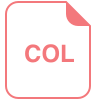
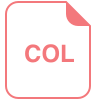
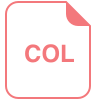
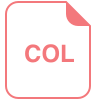
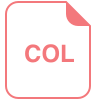
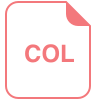
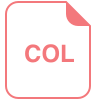
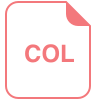
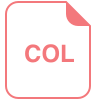
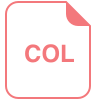
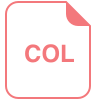
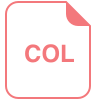