选择算法c++进行模板份文件编写代码
时间: 2023-08-09 13:11:52 浏览: 87
当你想要将模板函数的实现与声明分离到不同的文件中时,可以使用模板的显式实例化来实现模板函数的分文件编写。下面是一个示例,演示如何使用模板将选择排序算法的实现和声明分离到不同的文件中:
在`selectionsort.h`头文件中,声明选择排序算法的模板函数:
```cpp
#ifndef SELECTIONSORT_H
#define SELECTIONSORT_H
#include <vector>
template<typename T>
void selectionSort(std::vector<T>& arr);
#include "selectionsort.cpp" // 包含模板函数的实现
#endif
```
在`selectionsort.cpp`源文件中,实现选择排序算法的模板函数:
```cpp
#include "selectionsort.h"
template<typename T>
void selectionSort(std::vector<T>& arr) {
int n = arr.size();
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
std::swap(arr[i], arr[minIndex]);
}
}
// 显式实例化模板函数
template void selectionSort<int>(std::vector<int>& arr);
template void selectionSort<double>(std::vector<double>& arr);
// 可以根据需要添加其他类型的实例化
```
在主程序文件`main.cpp`中,使用选择排序算法进行排序:
```cpp
#include <iostream>
#include <vector>
#include "selectionsort.h"
int main() {
std::vector<int> nums = {5, 2, 8, 9, 1};
selectionSort(nums);
std::cout << "排序结果: ";
for (const auto& num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
在上面的示例中,`selectionsort.h`头文件中声明了选择排序算法的模板函数,并包含了`selectionsort.cpp`源文件,其中包含了模板函数的实现。
在`selectionsort.cpp`源文件中,我们使用了显式实例化来实例化选择排序算法的模板函数,以便在链接时能够生成对应类型的函数定义。这里示范了对`int`和`double`类型的实例化,你可以根据需要添加其他类型的实例化。
在主程序文件`main.cpp`中,我们包含了`selectionsort.h`头文件,并使用选择排序算法对向量进行排序。
请注意,在使用分文件编写模板时,需要将模板函数的实现放在`selectionsort.cpp`源文件中,并在需要使用模板函数的地方进行显式实例化。这样,在编译和链接时,编译器可以找到模板函数的实现并生成对应类型的函数定义。
希望这个示例能满足你的需求!如果还有其他问题,请随时提问。
阅读全文
相关推荐
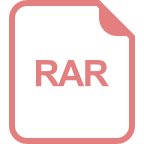
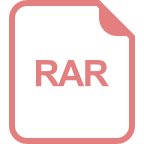
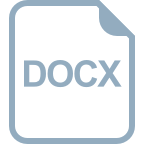
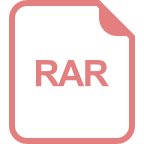
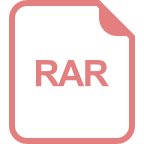
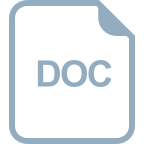
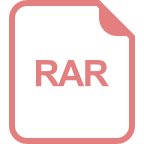
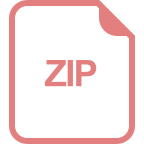
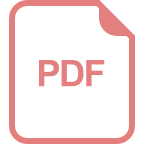
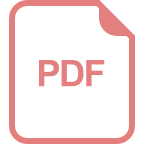
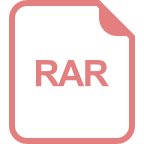
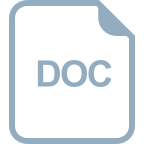
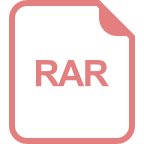
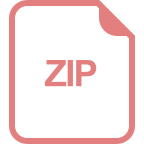
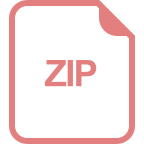