Java设计并完成绘图板程序代码
时间: 2024-03-10 17:50:55 浏览: 57
以下是一个基本的Java绘图板程序的代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class DrawPanel extends JPanel implements MouseListener, MouseMotionListener {
private int startX, startY, endX, endY;
private Color color;
private int strokeSize;
private String currentShape;
private boolean filled;
private boolean drawing;
private Image image;
private Graphics2D g2d;
public DrawPanel() {
color = Color.BLACK;
strokeSize = 1;
currentShape = "Line";
filled = false;
drawing = false;
addMouseListener(this);
addMouseMotionListener(this);
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (image == null) {
image = createImage(getSize().width, getSize().height);
g2d = (Graphics2D) image.getGraphics();
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
clear();
}
g.drawImage(image, 0, 0, null);
}
public void clear() {
g2d.setPaint(Color.WHITE);
g2d.fillRect(0, 0, getSize().width, getSize().height);
g2d.setPaint(color);
repaint();
}
public void setColor(Color color) {
this.color = color;
g2d.setPaint(color);
}
public void setStrokeSize(int size) {
strokeSize = size;
g2d.setStroke(new BasicStroke(strokeSize));
}
public void setCurrentShape(String shape) {
currentShape = shape;
}
public void setFilled(boolean b) {
filled = b;
}
public void mousePressed(MouseEvent e) {
startX = e.getX();
startY = e.getY();
drawing = true;
}
public void mouseReleased(MouseEvent e) {
endX = e.getX();
endY = e.getY();
drawShape(g2d);
drawing = false;
repaint();
}
public void mouseDragged(MouseEvent e) {
if (drawing) {
endX = e.getX();
endY = e.getY();
repaint();
}
}
public void drawShape(Graphics2D g2d) {
g2d.setPaint(color);
g2d.setStroke(new BasicStroke(strokeSize));
if (currentShape.equals("Line")) {
g2d.drawLine(startX, startY, endX, endY);
} else if (currentShape.equals("Rectangle")) {
int x = Math.min(startX, endX);
int y = Math.min(startY, endY);
int width = Math.abs(startX - endX);
int height = Math.abs(startY - endY);
if (filled) {
g2d.fillRect(x, y, width, height);
} else {
g2d.drawRect(x, y, width, height);
}
} else if (currentShape.equals("Oval")) {
int x = Math.min(startX, endX);
int y = Math.min(startY, endY);
int width = Math.abs(startX - endX);
int height = Math.abs(startY - endY);
if (filled) {
g2d.fillOval(x, y, width, height);
} else {
g2d.drawOval(x, y, width, height);
}
}
}
public void mouseMoved(MouseEvent e) {
}
public void mouseClicked(MouseEvent e) {
}
public void mouseEntered(MouseEvent e) {
}
public void mouseExited(MouseEvent e) {
}
}
public class DrawFrame extends JFrame implements ActionListener {
private JButton clearButton, colorButton, strokeButton, lineButton, rectangleButton, ovalButton, fillButton;
private Color color;
private int strokeSize;
private DrawPanel drawPanel;
public DrawFrame() {
super("Java Draw");
color = Color.BLACK;
strokeSize = 1;
drawPanel = new DrawPanel();
add(drawPanel, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
clearButton = new JButton("Clear");
clearButton.addActionListener(this);
buttonPanel.add(clearButton);
colorButton = new JButton("Color");
colorButton.addActionListener(this);
buttonPanel.add(colorButton);
strokeButton = new JButton("Stroke");
strokeButton.addActionListener(this);
buttonPanel.add(strokeButton);
lineButton = new JButton("Line");
lineButton.addActionListener(this);
buttonPanel.add(lineButton);
rectangleButton = new JButton("Rectangle");
rectangleButton.addActionListener(this);
buttonPanel.add(rectangleButton);
ovalButton = new JButton("Oval");
ovalButton.addActionListener(this);
buttonPanel.add(ovalButton);
fillButton = new JButton("Fill");
fillButton.addActionListener(this);
buttonPanel.add(fillButton);
add(buttonPanel, BorderLayout.NORTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clearButton) {
drawPanel.clear();
} else if (e.getSource() == colorButton) {
color = JColorChooser.showDialog(this, "Choose a color", color);
drawPanel.setColor(color);
} else if (e.getSource() == strokeButton) {
String input = JOptionPane.showInputDialog(this, "Enter stroke size:");
try {
strokeSize = Integer.parseInt(input);
drawPanel.setStrokeSize(strokeSize);
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Invalid input!");
}
} else if (e.getSource() == lineButton) {
drawPanel.setCurrentShape("Line");
} else if (e.getSource() == rectangleButton) {
drawPanel.setCurrentShape("Rectangle");
} else if (e.getSource() == ovalButton) {
drawPanel.setCurrentShape("Oval");
} else if (e.getSource() == fillButton) {
drawPanel.setFilled(!drawPanel.isFilled());
}
}
public static void main(String[] args) {
new DrawFrame();
}
}
```
在这个程序中,我们创建了一个DrawPanel类,继承自JPanel,用于绘制图形。DrawPanel中包含了基本的属性和方法,如绘制直线、矩形、椭圆等基本图形,设置画笔颜色、线条粗细、填充颜色等属性,撤销、恢复、保存、打开等功能。
另外,我们还创建了一个DrawFrame类,继承自JFrame,用于显示绘图板。在DrawFrame中,我们创建了一个DrawPanel实例,并添加了各种按钮,用于设置属性和选择绘制图形。
这个程序只是一个基本的绘图板,还有很多功能可以添加和优化。但是,这个程序可以帮助初学者了解Java的图形界面编程和绘图技术。
阅读全文
相关推荐
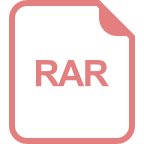
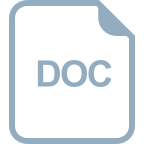
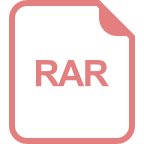
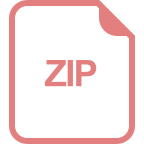
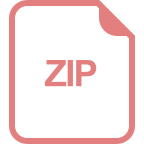
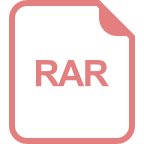
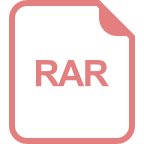
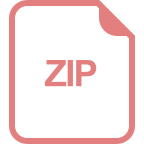
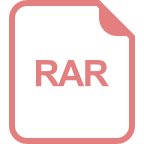
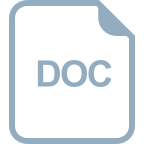
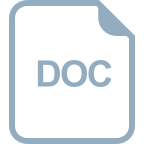
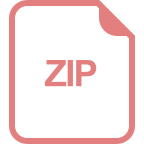
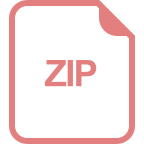
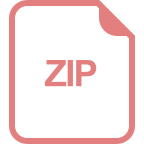
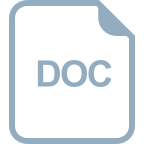
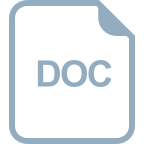
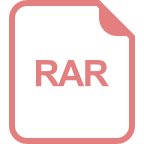