编写代码:QT按下按钮A打开线程执行循环命令,再按一次结束循环,结束线程。代码和构造函数在同一cpp文件中
时间: 2024-03-20 17:40:37 浏览: 25
下面是一个简单的示例代码,可以实现在Qt中按下按钮打开线程执行循环命令,并在再次按下相同按钮时结束循环和线程。
首先,我们需要在头文件中声明一个类来执行循环命令:
```c++
class MyThread : public QThread
{
Q_OBJECT
public:
MyThread(QObject* parent = 0);
void run();
void stop();
private:
bool m_running;
};
```
然后,在实现文件中,我们需要实现该类的构造函数、run()函数和stop()函数:
```c++
#include "mythread.h"
MyThread::MyThread(QObject* parent)
: QThread(parent)
{
m_running = false;
}
void MyThread::run()
{
m_running = true;
while (m_running)
{
// 循环命令的代码
}
}
void MyThread::stop()
{
m_running = false;
}
```
最后,在窗口类的构造函数中,我们可以连接按钮的clicked()信号到一个槽函数,该槽函数会在按钮被按下时创建一个MyThread对象并启动线程,在按钮再次被按下时停止线程:
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "mythread.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
MyThread* thread = new MyThread(this);
connect(ui->pushButton, &QPushButton::clicked, [=](){
if (!thread->isRunning())
{
thread->start();
ui->pushButton->setText("Stop");
}
else
{
thread->stop();
thread->wait();
ui->pushButton->setText("Start");
}
});
}
MainWindow::~MainWindow()
{
delete ui;
}
```
这样,当我们按下按钮时,会创建一个MyThread对象并启动线程,在线程中执行循环命令。再次按下按钮时,会停止线程并结束循环。
相关推荐
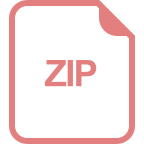
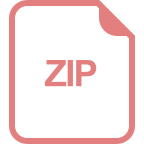














