图片压缩算法 c语言代码
时间: 2023-06-06 20:02:32 浏览: 127
图片压缩算法是一种将图像数据压缩为更小的数据量以节省存储空间或传输带宽的技术。下面是用C语言实现图片压缩算法的代码:
// 定义图像数据结构
typedef struct {
int width;
int height;
int channels;
unsigned char *data;
} Image;
// 算法实现
void compress(Image *image, int compressRatio) {
int i, j, k;
int newWidth = image->width / compressRatio;
int newHeight = image->height / compressRatio;
unsigned char *newData = (unsigned char*)malloc(newWidth * newHeight * image->channels);
for (i = 0; i < newHeight; i++) {
for (j = 0; j < newWidth; j++) {
for (k = 0; k < image->channels; k++) {
int sum = 0;
int count = compressRatio * compressRatio;
int x, y;
for (y = 0; y < compressRatio; y++) {
for (x = 0; x < compressRatio; x++) {
sum += image->data[((i * compressRatio + y) * image->width + j * compressRatio + x) * image->channels + k];
}
}
newData[(i * newWidth + j) * image->channels + k] = sum / count;
}
}
}
free(image->data);
image->data = newData;
image->width = newWidth;
image->height = newHeight;
}
上述代码实现了一种简单的图片压缩算法,它采用均值采样的方法,将原先的图像分成若干个小块,每个小块中的像素值取均值,得到新的块,从而达到图像压缩的目的。代码中compressRatio参数表示压缩比率,例如如果取2,则压缩后的图像尺寸为原来的一半。
相关推荐
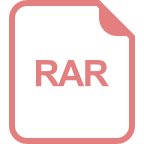














