用c++编写一个在vector容器中判断某个数是否存在的代码
时间: 2023-05-11 09:03:45 浏览: 131
以下是用 C++ 编写一个在 vector 容器中判断某个数是否存在的代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> nums = {1, 2, 3, 4, 5};
int target = 3;
if (find(nums.begin(), nums.end(), target) != nums.end()) {
cout << "Target exists in the vector." << endl;
} else {
cout << "Target does not exist in the vector." << endl;
}
return 0;
}
```
这段代码中,我们首先定义了一个 vector 容器 nums,其中包含了一些整数。然后我们定义了一个目标数 target,我们需要判断这个数是否存在于 nums 中。
我们使用了 C++ 标准库中的 find 函数来查找 target 是否存在于 nums 中。如果 find 函数返回的迭代器不等于 nums.end(),则说明 target 存在于 nums 中,否则说明 target 不存在于 nums 中。
注意:这段代码只是一个示例,实际使用时需要根据具体情况进行修改。
相关问题
给我编一段实现将C+++中vector容器进行快速排序并打印的代码
如下是一个使用 C++ 的 STL 中的 std::sort 函数对 vector 容器进行快速排序,并将排序结果打印出来的示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> vec = {3, 1, 4, 2, 5}; // 初始化一个包含 5 个元素的 vector 容器
sort(vec.begin(), vec.end()); // 调用 sort 函数对 vec 进行快速排序
for (auto it = vec.begin(); it != vec.end(); it++) {
cout << *it << " "; // 打印排序结果
}
return 0;
}
```
请注意,这段代码使用了 C++ 的 STL 中的 sort 函数,如果您想使用纯 C 的代码实现快速排序,则需要自己编写快速排序算法。
用c++编写一段产生20个100至999之间的随机数并存放到vector容器中
可以使用以下代码实现:
```c++
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
vector<int> nums;
srand(time(NULL)); // 设置随机数种子
for (int i = 0; i < 20; i++) {
int num = rand() % 900 + 100; // 生成100-999之间的随机数
nums.push_back(num); // 将随机数添加到vector容器中
}
// 输出vector容器中的随机数
for (int i = 0; i < nums.size(); i++) {
cout << nums[i] << " ";
}
cout << endl;
return 0;
}
```
这段代码使用了rand()函数生成随机数,srand()函数设置了随机数种子,保证每次运行程序生成的随机数不同。使用vector容器存储随机数,最后输出vector容器中的随机数。
阅读全文
相关推荐
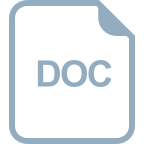
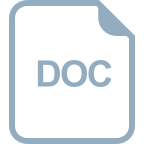
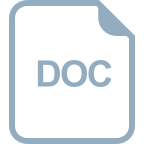
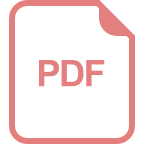
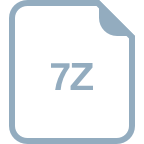
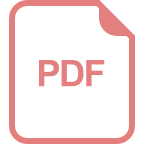
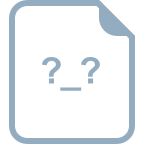
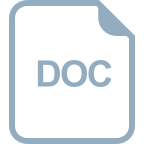
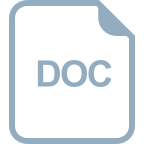
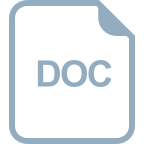
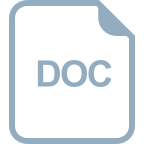
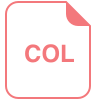
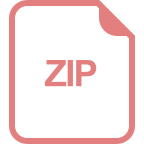
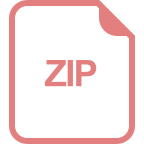
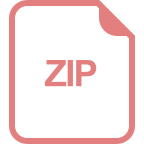
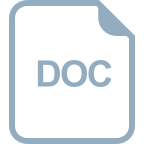
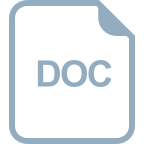