python怎么找出列表中出现次数最多的数
时间: 2023-08-08 08:13:35 浏览: 106
在 Python 中,可以使用 collections 模块中的 Counter 类来统计列表中每个元素出现的次数,然后使用 most_common() 方法找出出现次数最多的元素和它的出现次数。
下面是一个示例代码:
```python
from collections import Counter
my_list = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1]
counter = Counter(my_list)
most_common = counter.most_common(1)
print(most_common[0][0])
```
运行结果:
```
1
```
这个示例中,my_list 是一个包含了一些整数的列表,我们使用 Counter 类来统计每个元素出现的次数,然后使用 most_common() 方法找出出现次数最多的元素和它的出现次数。由于我们只需要找出出现次数最多的元素,因此将 most_common() 方法的参数设置为 1。最后,使用 print() 函数打印出出现次数最多的元素。
相关问题
在python中怎么找出列表中出现次数最多的数
在Python中,可以使用collections模块中的Counter函数来统计列表中每个元素出现的次数,并使用most_common()函数返回出现次数最多的元素和它的出现次数。具体实现如下:
```python
from collections import Counter
my_list = [1, 2, 3, 4, 5, 1, 2, 3, 1, 2, 1]
counter = Counter(my_list)
most_common = counter.most_common(1)
print(most_common[0][0])
```
输出结果为:
```
1
```
表示列表中出现次数最多的数是1。
python如何找出数组中出现最多的数据
在Python中,你可以使用collections模块中的Counter类或者直接使用内置函数来找出数组(列表)中出现次数最多的元素。以下是两种常见方法:
1. 使用`collections.Counter`:
```python
from collections import Counter
def find_most_common(lst):
counter = Counter(lst)
return counter.most_common(1)[0][0]
# 示例
numbers = [1, 2, 3, 4, 4, 4, 5]
most_common_num = find_most_common(numbers)
print(f"出现最多的数是:{most_common_num}")
```
2. 直接遍历并计数:
```python
def find_most_common(lst):
max_count = 0
most_common = None
for num in lst:
count = lst.count(num)
if count > max_count:
max_count = count
most_common = num
return most_common
numbers = [1, 2, 3, 4, 4, 4, 5]
most_common_num = find_most_common(numbers)
print(f"出现最多的数是:{most_common_num}")
```
在这两个例子中,返回的结果将是出现次数最多的元素及其出现的次数。如果你想只得到元素,可以稍微修改一下函数。
阅读全文
相关推荐
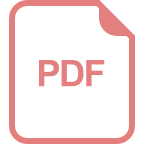
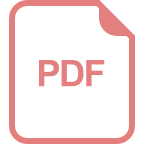
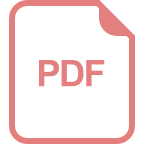
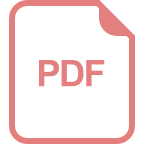
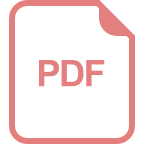










