Lvgl sd卡文件查看器的代码
时间: 2024-01-22 15:02:17 浏览: 255
以下是基于LVGL的SD卡文件查看器的代码示例:
```c
#include "lvgl/lvgl.h"
#include "lvgl_helpers.h"
#include "ff.h"
#include <stdio.h>
#include <string.h>
#define MAX_PATH_LEN 128
#define MAX_FILE_NAME_LEN 32
#define MAX_FILE_SIZE 1024
/* UI elements */
static lv_obj_t *file_list;
static lv_obj_t *file_name_label;
static lv_obj_t *file_content_label;
/* File system */
static FATFS fs;
static DIR dir;
static FILINFO fno;
static FIL file;
/* Buffers */
static char path_buf[MAX_PATH_LEN];
static char file_name_buf[MAX_FILE_NAME_LEN];
static char file_content_buf[MAX_FILE_SIZE];
/* Current state */
static char current_dir[MAX_PATH_LEN];
static char current_file[MAX_FILE_NAME_LEN];
/* List of supported file extensions */
static const char *supported_extensions[] = {".txt", ".log", ".cfg", NULL};
/* Forward declaration of functions */
static void list_files(const char *path);
/* Callback for file list */
static lv_res_t file_list_cb(lv_obj_t *list, const char *txt)
{
lv_obj_set_hidden(file_content_label, true);
if (txt[0] == '/')
{
/* Open new directory */
if (f_opendir(&dir, txt) != FR_OK)
{
lv_label_set_text(file_name_label, "[Error]");
return LV_RES_OK;
}
strcpy(current_dir, txt);
list_files(current_dir);
}
else
{
/* Open file */
snprintf(path_buf, sizeof(path_buf), "%s/%s", current_dir, txt);
if (f_open(&file, path_buf, FA_READ) != FR_OK)
{
lv_label_set_text(file_name_label, "[Error]");
return LV_RES_OK;
}
strcpy(current_file, txt);
/* Read file content */
UINT bytes_read = 0;
f_read(&file, file_content_buf, sizeof(file_content_buf), &bytes_read);
file_content_buf[bytes_read] = '\0';
/* Set UI elements */
lv_label_set_text(file_name_label, current_file);
lv_label_set_text(file_content_label, file_content_buf);
lv_obj_set_hidden(file_content_label, false);
}
return LV_RES_OK;
}
/* List files in directory */
static void list_files(const char *path)
{
lv_obj_clean(file_list);
/* Add parent directory */
if (strcmp(path, "/") != 0)
{
lv_obj_t *parent = lv_list_add(file_list, LV_SYMBOL_DIRECTORY, "..");
lv_obj_set_event_cb(parent, file_list_cb);
}
/* Open directory */
if (f_opendir(&dir, path) != FR_OK)
{
lv_label_set_text(file_name_label, "[Error]");
return;
}
/* Iterate over directory entries */
while (f_readdir(&dir, &fno) == FR_OK && fno.fname[0] != 0)
{
if (fno.fattrib & AM_DIR)
{
/* Add directory */
lv_obj_t *dir_obj = lv_list_add(file_list, LV_SYMBOL_DIRECTORY, fno.fname);
snprintf(path_buf, sizeof(path_buf), "%s/%s", path, fno.fname);
lv_obj_set_event_cb(dir_obj, file_list_cb);
}
else
{
/* Check if file extension is supported */
const char *ext = strrchr(fno.fname, '.');
if (ext != NULL && strcasecmp(ext, ".txt") == 0)
{
/* Add file */
lv_obj_t *file_obj = lv_list_add(file_list, LV_SYMBOL_FILE, fno.fname);
lv_obj_set_event_cb(file_obj, file_list_cb);
}
}
}
f_closedir(&dir);
}
/* Initialize UI */
static void init_ui(lv_obj_t *parent)
{
/* Create file list */
file_list = lv_list_create(parent, NULL);
lv_obj_set_size(file_list, LV_HOR_RES - 20, LV_VER_RES - 100);
lv_obj_align(file_list, NULL, LV_ALIGN_IN_TOP_LEFT, 10, 10);
/* Create file name label */
file_name_label = lv_label_create(parent, NULL);
lv_label_set_text(file_name_label, "");
lv_obj_align(file_name_label, file_list, LV_ALIGN_OUT_BOTTOM_LEFT, 0, 10);
/* Create file content label */
file_content_label = lv_label_create(parent, NULL);
lv_label_set_text(file_content_label, "");
lv_obj_set_size(file_content_label, LV_HOR_RES - 20, LV_VER_RES - 100);
lv_obj_align(file_content_label, file_list, LV_ALIGN_IN_TOP_LEFT, 10, 10);
lv_obj_set_hidden(file_content_label, true);
}
/* Initialize file system */
static void init_fs()
{
f_mount(&fs, "", 0);
list_files("/");
}
int main(int argc, char **argv)
{
/* Initialize LVGL */
lv_init();
/* Initialize display */
lvgl_driver_init();
/* Create main screen */
lv_obj_t *scr = lv_obj_create(NULL, NULL);
lv_scr_load(scr);
/* Initialize UI */
init_ui(scr);
/* Initialize file system */
init_fs();
/* Event loop */
while (1)
{
lv_task_handler();
SDL_Delay(5);
}
return 0;
}
```
这是一个简单的SD卡文件查看器,它使用LVGL作为UI库,FatFs作为文件系统库。该程序支持打开.txt、.log、.cfg等文件类型。在UI上,它使用了一个列表来显示当前目录的文件和子目录,选择文件后会在UI上显示文件名和内容。
阅读全文
相关推荐




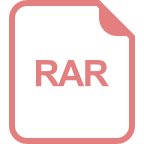
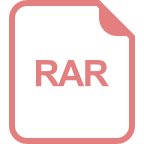



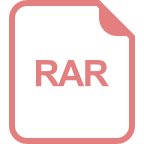



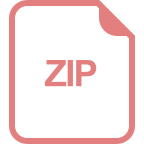



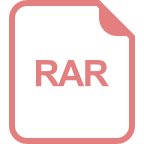