我需要对bmp图像进行处理,并且更简单的代码
时间: 2024-03-05 20:54:07 浏览: 23
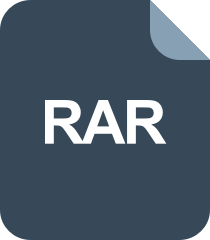
bmp图像的简单处理
下面是一个简单的C语言代码,用于对BMP图像进行直方图均衡化处理:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define WIDTHBYTES(bits) (((bits) + 31) / 32 * 4)
void histogram_equalization(unsigned char *img, int width, int height);
int main(int argc, char *argv[])
{
if (argc != 2)
{
printf("Usage: %s image.bmp\n", argv[0]);
return 1;
}
FILE *fp = fopen(argv[1], "rb");
if (!fp)
{
printf("Failed to open file: %s\n", argv[1]);
return 1;
}
unsigned char header[54];
fread(header, sizeof(unsigned char), 54, fp);
int width = *(int *)(header + 18);
int height = *(int *)(header + 22);
int bits_per_pixel = *(unsigned short *)(header + 28);
if (bits_per_pixel != 24)
{
printf("Only support 24bit BMP image\n");
fclose(fp);
return 1;
}
int row_size = WIDTHBYTES(width * bits_per_pixel);
unsigned char *img = malloc(row_size * height);
fread(img, sizeof(unsigned char), row_size * height, fp);
fclose(fp);
histogram_equalization(img, width, height);
fp = fopen("output.bmp", "wb");
if (!fp)
{
printf("Failed to create output file\n");
free(img);
return 1;
}
fwrite(header, sizeof(unsigned char), 54, fp);
fwrite(img, sizeof(unsigned char), row_size * height, fp);
fclose(fp);
free(img);
return 0;
}
void histogram_equalization(unsigned char *img, int width, int height)
{
int hist[256] = {0};
int cdf[256] = {0};
int pixel_count = width * height;
for (int i = 0; i < pixel_count; i++)
{
int gray = 0.299 * img[3 * i + 2] + 0.587 * img[3 * i + 1] + 0.114 * img[3 * i];
hist[gray]++;
}
cdf[0] = hist[0];
for (int i = 1; i < 256; i++)
{
cdf[i] = cdf[i - 1] + hist[i];
}
for (int i = 0; i < pixel_count; i++)
{
int gray = 0.299 * img[3 * i + 2] + 0.587 * img[3 * i + 1] + 0.114 * img[3 * i];
img[3 * i] = img[3 * i + 1] = img[3 * i + 2] =
(unsigned char)(cdf[gray] * 255.0 / pixel_count + 0.5);
}
}
```
该代码实现了对24位BMP图像的直方图均衡化,基本思路是读取BMP图像,然后计算图像灰度直方图,接着计算累计分布函数(CDF),最后根据CDF对图像进行均衡化处理。该代码使用了24位BMP图像的RGB通道进行灰度计算,处理后的图像保存为output.bmp文件。
阅读全文
相关推荐
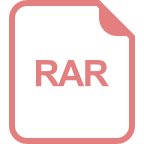
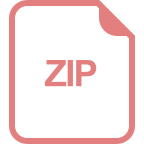
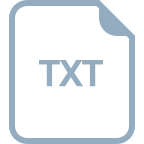
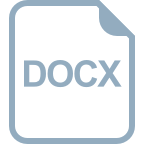
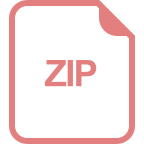