(1) 计费功能。根据存放在源数据文件中的通话记录和长途费率文件对每一条通话记录计算其通话费用,并将结果保存在费用文件中。其中: 通话费的计算方法如下: 通话费=长途电话费+本地电话费 长途电话费=费率(元/分钟)×通话时长(分钟) (通话时长不满1分钟的按1分钟计算) 本地电话费为:3分钟以内0.5元,以后每3分钟递增0.2元。 (2) 话费查询。输入一个电话号码,从费用文件中统计该电话号码的所有本地话费、长途话费,并从用户文件中查找其用户名,最后在屏幕上显示: 用户名 电话号码 本地话费 长途话费 话费总计 (3) 话单查询。输入一个电话号码,查询并在屏幕显示该用户的所有通话记录,格式为: 用户名 主叫电话号码 被叫电话号码 通话时长
时间: 2024-03-30 21:39:15 浏览: 216
这是一个比较复杂的程序,需要涉及到文件的读写和数据的处理。以下是一个简单的实现思路:
1. 定义数据结构
我们需要定义三个数据结构:通话记录结构体、用户信息结构体和费用信息结构体。通话记录结构体包含主叫和被叫电话号码、通话时长等信息;用户信息结构体包含用户名和电话号码等信息;费用信息结构体包含本地话费、长途话费和总话费等信息。
```cpp
// 通话记录结构体
struct CallRecord {
string caller; // 主叫电话号码
string callee; // 被叫电话号码
int duration; // 通话时长(单位:秒)
};
// 用户信息结构体
struct UserInfo {
string name; // 用户名
string phone; // 电话号码
};
// 费用信息结构体
struct CostInfo {
double localCost; // 本地话费
double longDistanceCost; // 长途话费
double totalCost; // 总话费
};
```
2. 读取源数据文件和长途费率文件
我们需要读取存放通话记录的源数据文件和长途费率文件。通话记录文件中每行包含主叫号码、被叫号码和通话时长,以逗号分隔;长途费率文件中每行包含地区代码和费率,以逗号分隔。
```cpp
// 读取通话记录文件
vector<CallRecord> readCallRecords(string filename) {
vector<CallRecord> records;
ifstream fin(filename);
if (!fin) {
cout << "Failed to open file " << filename << endl;
return records;
}
string line;
while (getline(fin, line)) {
stringstream ss(line);
string caller, callee;
int duration;
getline(ss, caller, ',');
getline(ss, callee, ',');
ss >> duration;
records.push_back({ caller, callee, duration });
}
fin.close();
return records;
}
// 读取长途费率文件
map<string, double> readLongDistanceRates(string filename) {
map<string, double> rates;
ifstream fin(filename);
if (!fin) {
cout << "Failed to open file " << filename << endl;
return rates;
}
string line;
while (getline(fin, line)) {
stringstream ss(line);
string code;
double rate;
getline(ss, code, ',');
ss >> rate;
rates[code] = rate;
}
fin.close();
return rates;
}
```
3. 计算通话费用
我们使用上面读取到的通话记录和长途费率,计算每条通话记录的通话费用,并将结果保存在费用文件中。本地话费和长途话费分别计算,然后相加得到总话费。
```cpp
// 计算通话费用
CostInfo calculateCost(const CallRecord& record, const map<string, double>& rates) {
CostInfo cost;
// 计算长途话费
string code = record.callee.substr(0, 3);
double rate = rates[code];
int minutes = (record.duration + 59) / 60; // 不足1分钟按1分钟计算
cost.longDistanceCost = rate * minutes;
// 计算本地话费
int localMinutes = (record.duration + 2) / 60; // 向上取整到分钟
if (localMinutes <= 3) {
cost.localCost = 0.5;
} else {
cost.localCost = 0.5 + (localMinutes - 3) / 3 * 0.2;
}
// 计算总话费
cost.totalCost = cost.longDistanceCost + cost.localCost;
return cost;
}
// 计算所有通话记录的费用并保存到文件中
void calculateAllCosts(const vector<CallRecord>& records, const map<string, double>& rates, string filename) {
ofstream fout(filename);
if (!fout) {
cout << "Failed to open file " << filename << endl;
return;
}
for (const CallRecord& record : records) {
CostInfo cost = calculateCost(record, rates);
fout << record.caller << "," << record.callee << "," << record.duration << "," << cost.localCost << "," << cost.longDistanceCost << "," << cost.totalCost << endl;
}
fout.close();
}
```
4. 话费查询
我们输入一个电话号码,从费用文件中统计该电话号码的所有本地话费、长途话费,并从用户文件中查找其用户名,最后在屏幕上显示用户名、电话号码、本地话费、长途话费和总话费。
```cpp
// 查询话费
void queryCost(const string& phone, const string& userFilename, const string& costFilename) {
// 查找用户名
string name;
ifstream fin(userFilename);
if (!fin) {
cout << "Failed to open file " << userFilename << endl;
return;
}
string line;
while (getline(fin, line)) {
stringstream ss(line);
string _name, _phone;
getline(ss, _name, ',');
getline(ss, _phone, ',');
if (_phone == phone) {
name = _name;
break;
}
}
fin.close();
// 统计本地话费、长途话费和总话费
double localCost = 0, longDistanceCost = 0, totalCost = 0;
ifstream fin2(costFilename);
if (!fin2) {
cout << "Failed to open file " << costFilename << endl;
return;
}
while (getline(fin2, line)) {
stringstream ss(line);
string caller, callee;
int duration;
double _localCost, _longDistanceCost, _totalCost;
getline(ss, caller, ',');
getline(ss, callee, ',');
ss >> duration >> _localCost >> _longDistanceCost >> _totalCost;
if (caller == phone) {
localCost += _localCost;
longDistanceCost += _longDistanceCost;
totalCost += _totalCost;
}
}
fin2.close();
// 输出结果
cout << name << " " << phone << " " << localCost << " " << longDistanceCost << " " << totalCost << endl;
}
```
5. 话单查询
我们输入一个电话号码,查询并在屏幕显示该用户的所有通话记录,格式为用户名、主叫电话号码、被叫电话号码和通话时长。
```cpp
// 查询话单
void queryCallRecords(const string& phone, const string& userFilename, const string& recordFilename) {
// 查找用户名
string name;
ifstream fin(userFilename);
if (!fin) {
cout << "Failed to open file " << userFilename << endl;
return;
}
string line;
while (getline(fin, line)) {
stringstream ss(line);
string _name, _phone;
getline(ss, _name, ',');
getline(ss, _phone, ',');
if (_phone == phone) {
name = _name;
break;
}
}
fin.close();
// 输出通话记录
ifstream fin2(recordFilename);
if (!fin2) {
cout << "Failed to open file " << recordFilename << endl;
return;
}
while (getline(fin2, line)) {
stringstream ss(line);
string caller, callee;
int duration;
getline(ss, caller, ',');
getline(ss, callee, ',');
ss >> duration;
if (caller == phone || callee == phone) {
if (caller == phone) {
cout << name << " " << caller << " " << callee << " " << duration << endl;
} else {
cout << name << " " << callee << " " << caller << " " << duration << endl;
}
}
}
fin2.close();
}
```
完整的程序如下:
阅读全文
相关推荐
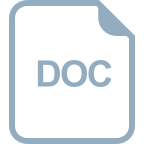
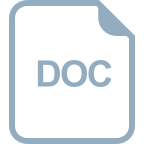
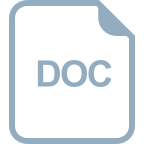

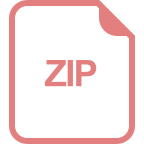
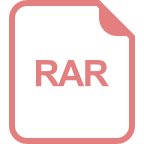
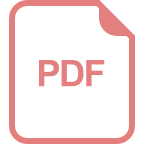
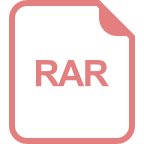
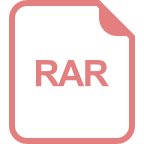
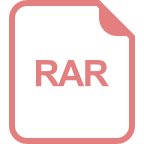
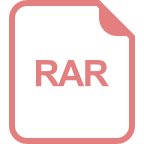
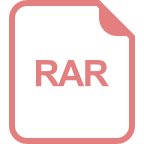
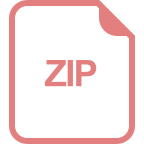
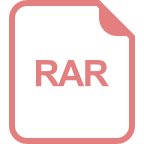
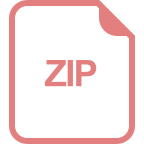
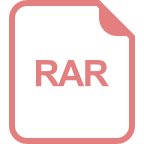
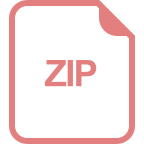
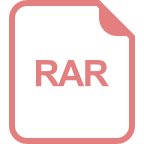