后端怎么接收使用autocomplete输入地址的前端 接着返送到google地图上并显示最近的停车场 使用javascript写代码
时间: 2024-05-13 15:20:34 浏览: 114
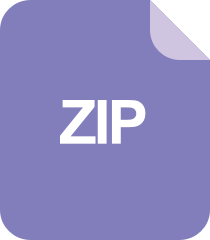
google-place-autocomplete:React在React上使用Google Place Autocomplete API的最佳做法
后端可以通过提供一个API接口来接收前端输入的地址信息,并将其发送到Google地图API服务端进行解析和查询。查询结果包括该地址周围的停车场信息,后端再将这些信息返回给前端进行展示。
以下是一个简单的JavaScript代码示例,用于向后端API发送地址信息并获取返回的停车场信息:
```javascript
// 获取地址输入框元素
const addressInput = document.getElementById('address-input');
// 监听地址输入框的键盘输入事件
addressInput.addEventListener('keyup', async (e) => {
// 如果输入的是回车键
if (e.keyCode === 13) {
// 发送地址信息到后端API
const response = await fetch('/api/parking-lots', {
method: 'POST',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify({address: addressInput.value})
});
// 解析API返回的JSON数据
const data = await response.json();
// 在页面上展示停车场信息
const parkingLots = data.parkingLots;
// ...
}
});
```
在后端API中,可以使用Google Maps JavaScript API和Google Places API来查询指定地址周围的停车场信息。以下是一个简单的Node.js代码示例:
```javascript
const express = require('express');
const bodyParser = require('body-parser');
const {Client} = require('@googlemaps/google-maps-services-js');
const app = express();
const client = new Client({});
// 解析JSON请求体
app.use(bodyParser.json());
// 处理地址查询请求
app.post('/api/parking-lots', async (req, res) => {
try {
// 调用Google Maps API获取地理编码信息
const geocodeResponse = await client.geocode({
params: {address: req.body.address},
timeout: 1000 // 超时时间1秒
});
if (geocodeResponse.data.status !== 'OK') {
throw new Error('Invalid address');
}
// 获取地理编码结果的经纬度
const location = geocodeResponse.data.results[0].geometry.location;
// 调用Google Places API获取停车场信息
const placesResponse = await client.placesNearby({
params: {
location: `${location.lat},${location.lng}`,
radius: 500, // 搜索半径500米
type: 'parking', // 类型为停车场
key: 'YOUR_GOOGLE_MAPS_API_KEY'
},
timeout: 1000 // 超时时间1秒
});
// 将停车场信息返回给前端
res.json({parkingLots: placesResponse.data.results});
} catch (error) {
console.error(error);
res.status(500).json({error: error.message});
}
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
```
需要注意的是,上述示例中的`YOUR_GOOGLE_MAPS_API_KEY`需要替换为你自己的Google Maps API密钥。此外,Google Maps API和Google Places API都需要在Google Cloud Console中启用并开通相关服务。
阅读全文
相关推荐
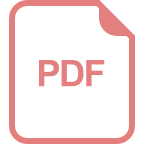
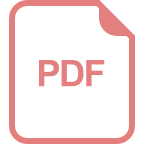















