A*算法源码的具体分析,定义节点类,评估函数
时间: 2023-07-20 11:06:55 浏览: 87
A*算法是一种基于启发式搜索的寻路算法,常用于游戏开发、机器人导航等领域。该算法通过评估每个节点的价值,来决定在搜索过程中应该先扩展哪些节点,从而找到最优解。
下面我们来看一下A*算法的具体实现过程:
## 1. 定义节点类
在A*算法中,我们需要定义一个节点类来表示搜索过程中的每个状态。节点类包含以下几个属性:
- `position`:节点的位置
- `parent`:父节点
- `g_score`:从起点到该节点的实际代价
- `h_score`:从该节点到终点的估计代价
- `f_score`:从起点到终点的估计代价
```python
class Node:
def __init__(self, position, parent=None, g_score=0, h_score=0):
self.position = position
self.parent = parent
self.g_score = g_score
self.h_score = h_score
self.f_score = g_score + h_score
```
## 2. 定义评估函数
A*算法中的评估函数通常称为启发函数(heuristic function),用来评估从当前节点到终点的代价。启发函数需要满足以下两个条件:
- 启发函数的值必须小于或等于从当前节点到终点的实际代价(即必须是一种可行的估计)。
- 启发函数的值越小,表示到达终点的代价越小,算法越有可能在搜索过程中选择该节点。
常用的启发函数包括曼哈顿距离、欧几里得距离、切比雪夫距离等。以曼哈顿距离为例,假设当前节点位置为`(x1, y1)`,终点位置为`(x2, y2)`,则从当前节点到终点的曼哈顿距离为:`abs(x1 - x2) + abs(y1 - y2)`。
```python
def manhattan_distance(position, goal):
return abs(position[0] - goal[0]) + abs(position[1] - goal[1])
```
## 3. 实现A*算法
实现A*算法的过程如下:
- 将起点添加到开放列表(open_list)中。
- 从开放列表中取出f_score最小的节点作为当前节点。
- 如果当前节点是终点,则返回路径。
- 否则,将当前节点从开放列表中移除,并将其添加到关闭列表(closed_list)中。
- 对当前节点的相邻节点进行扩展,计算它们的g_score、h_score和f_score,并将它们添加到开放列表中。
- 重复第2步至第5步,直到找到终点或者开放列表为空。
```python
def astar(start, goal, get_neighbors, heuristic):
# 初始化起点和终点
start_node = Node(start)
goal_node = Node(goal)
# 初始化开放列表和关闭列表
open_list = [start_node]
closed_list = []
while open_list:
# 取出f_score最小的节点作为当前节点
current_node = min(open_list, key=lambda node: node.f_score)
# 如果当前节点是终点,则返回路径
if current_node.position == goal_node.position:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
# 将当前节点从开放列表中移除,并将其添加到关闭列表中
open_list.remove(current_node)
closed_list.append(current_node)
# 对当前节点的相邻节点进行扩展
for neighbor_position in get_neighbors(current_node.position):
neighbor_node = Node(
neighbor_position,
parent=current_node,
g_score=current_node.g_score + 1,
h_score=heuristic(neighbor_position, goal_node.position)
)
# 如果相邻节点已经在关闭列表中,则跳过
if neighbor_node in closed_list:
continue
# 如果相邻节点不在开放列表中,则将其添加到开放列表中
if neighbor_node not in open_list:
open_list.append(neighbor_node)
else:
# 如果相邻节点已经在开放列表中,更新它的g_score和f_score
index = open_list.index(neighbor_node)
if neighbor_node.g_score < open_list[index].g_score:
open_list[index].g_score = neighbor_node.g_score
open_list[index].f_score = neighbor_node.f_score
# 如果搜索失败,则返回空路径
return []
```
以上就是A*算法的具体实现过程。在实际应用中,我们可以根据具体情况选择合适的启发函数,并使用该算法来寻找最优路径。
阅读全文
相关推荐
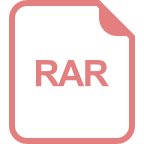
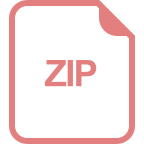
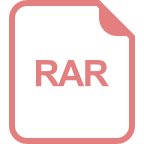
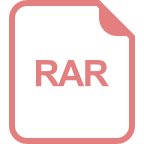
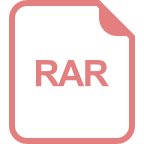
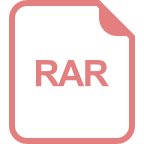
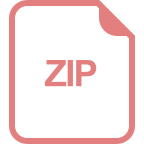
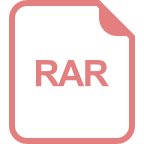
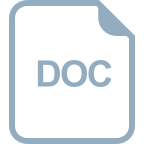
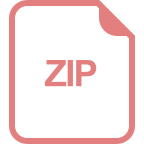
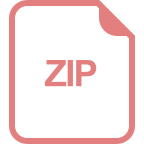
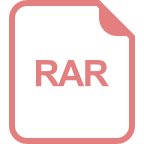
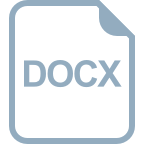
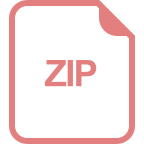
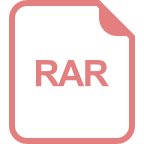
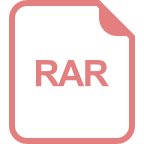