编写java程序,随便输入两个单词,两个单词之间以空格隔开,输出时每个单词的首字母变为大写。如输入:“hello java”,输出为“hello java”
时间: 2023-06-05 10:47:48 浏览: 140
以下是Java程序实现:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
String[] words = input.split(" ");
String output = "";
for (String word : words) {
output += word.substring(0, 1).toUpperCase() + word.substring(1) + " ";
}
System.out.println(output.trim());
}
}
运行程序后,输入两个单词,程序会输出每个单词的首字母变为大写的结果。
相关问题
编写一个Java程序,输入一个字符串并把每个单词的首字母大写。
以下是一个Java程序,可以实现输入一个字符串并把每个单词的首字母大写:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个字符串:");
String input = scanner.nextLine();
String[] words = input.split(" ");
StringBuilder result = new StringBuilder();
for (String word : words) {
result.append(word.substring(0, 1).toUpperCase()).append(word.substring(1)).append(" ");
}
System.out.println("首字母大写后的字符串为:" + result.toString().trim());
}
}
```
运行程序后,会提示用户输入一个字符串。程序会按照空格分隔输入的字符串,然后把每个单词的首字母大写后,再拼接成一个新的字符串输出。注意,最后输出的字符串末尾可能会有多余的空格,需要使用 `trim()` 方法去掉。
java输入一个字符串(单词之间以空格隔开),输出不重复的单词
可以使用Java中的HashMap来实现。
具体步骤如下:
1. 读取输入的字符串。
2. 将字符串按照空格分隔成单词,存入一个数组中。
3. 创建一个HashMap,遍历该数组,将每个单词作为key存入HashMap中,value设为1。
4. 最后遍历HashMap,输出所有key即可,这些key就是不重复的单词。
下面是示例代码:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine(); // 读取输入的字符串
String[] words = str.split(" "); // 将字符串按照空格分隔成单词,存入数组中
Map<String, Integer> map = new HashMap<>();
for (String word : words) {
map.put(word, 1); // 将每个单词作为key存入HashMap中,value设为1
}
// 遍历HashMap,输出所有key
for (String key : map.keySet()) {
System.out.print(key + " ");
}
}
}
```
相关推荐
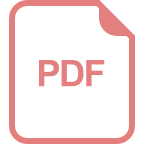
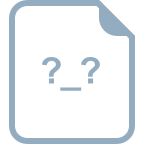












