用qt写一个网络聊天室
时间: 2023-09-03 21:07:31 浏览: 98
下面是一个简单的Qt网络聊天室的实现示例:
1. 创建一个Qt项目,选择"Qt Widgets Application"类型。
2. 在项目中添加一个MainWindow类,作为我们的主窗口。
3. 在MainWindow类中添加以下代码:
```cpp
#include <QtWidgets/QMainWindow>
#include <QtNetwork/QTcpSocket>
#include <QtNetwork/QTcpServer>
#include <QtWidgets/QTextEdit>
#include <QtWidgets/QLineEdit>
#include <QtWidgets/QPushButton>
#include <QtWidgets/QLabel>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = 0);
~MainWindow();
private slots:
void newConnection();
void readData();
void sendData();
private:
QTcpServer *server;
QTcpSocket *socket;
QTextEdit *textEdit;
QLineEdit *lineEdit;
QPushButton *sendButton;
QLabel *statusLabel;
};
```
4. 在MainWindow构造函数中初始化各个组件:
```cpp
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent) {
setWindowTitle("Chat Room");
textEdit = new QTextEdit(this);
textEdit->setReadOnly(true);
textEdit->setGeometry(0, 0, 400, 300);
lineEdit = new QLineEdit(this);
lineEdit->setGeometry(0, 310, 300, 30);
sendButton = new QPushButton("Send", this);
sendButton->setGeometry(310, 310, 90, 30);
statusLabel = new QLabel("Not connected", this);
statusLabel->setGeometry(0, 340, 400, 20);
server = new QTcpServer(this);
connect(server, SIGNAL(newConnection()), this, SLOT(newConnection()));
if (!server->listen(QHostAddress::Any, 1234)) {
statusLabel->setText("Failed to start server");
} else {
statusLabel->setText("Server started");
}
}
```
5. 在MainWindow的析构函数中清理资源:
```cpp
MainWindow::~MainWindow() {
delete textEdit;
delete lineEdit;
delete sendButton;
delete statusLabel;
delete server;
if (socket) {
delete socket;
}
}
```
6. 在newConnection()槽函数中处理新连接:
```cpp
void MainWindow::newConnection() {
socket = server->nextPendingConnection();
connect(socket, SIGNAL(readyRead()), this, SLOT(readData()));
statusLabel->setText("Connected");
}
```
7. 在readData()槽函数中处理接收到的数据:
```cpp
void MainWindow::readData() {
QByteArray data = socket->readAll();
textEdit->append(QString(data));
}
```
8. 在sendData()槽函数中发送数据:
```cpp
void MainWindow::sendData() {
if (socket) {
QString text = lineEdit->text();
socket->write(text.toUtf8());
textEdit->append(QString("Me: ") + text);
lineEdit->setText("");
}
}
```
9. 最后,在MainWindow的构造函数中连接sendButton的clicked()信号到sendData()槽函数:
```cpp
connect(sendButton, SIGNAL(clicked()), this, SLOT(sendData()));
```
现在,我们的网络聊天室就完成了!运行程序,打开两个窗口,一个作为服务器,一个作为客户端,就可以相互发送消息了。
阅读全文
相关推荐
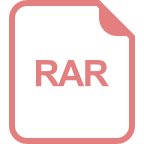

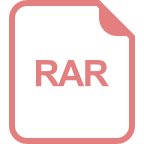
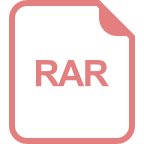
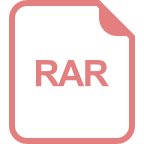
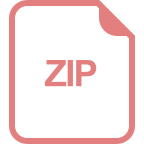
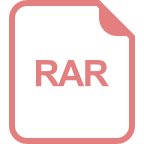
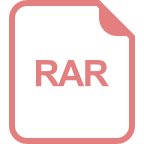
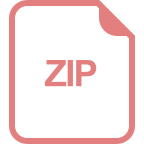
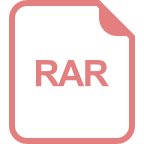
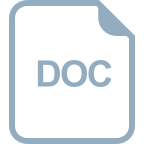
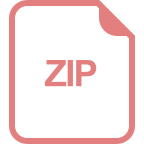
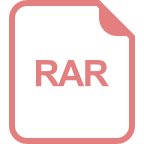
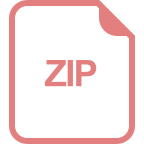