设计一个名为rectangle的类表示矩形。这个类包括: 两个名为width和height的double类型数据域,它们分别表示矩形的宽和高。width和height的默认值都为1。 一个用于创建默认矩形的无参构造方法。 一个创建指定width和height值的矩形的构造方法。 一个名为getarea()的方法,返回该矩形的面积。 一个名为getperimeter()的方法,返回周长。
时间: 2023-05-31 20:17:54 浏览: 183
### 回答1:
这是一个名为rectangle的类表示矩形。这个类包括:两个名为width和height的double类型数据域,它们分别表示矩形的宽和高。width和height的默认值都为1。一个用于创建默认矩形的无参构造方法。一个用于创建指定width和height值的构造方法。一个名为getArea()的方法,返回该矩形的面积。一个名为getPerimeter()的方法,返回该矩形的周长。
### 回答2:
首先定义一个名为Rectangle的类来表示矩形,类中包含两个double类型的数据域width和height,分别表示矩形的宽和高,它们的默认值都为1。我们可以采用以下代码来实现:
class Rectangle{
private:
double width = 1;
double height = 1;
public:
Rectangle(){}
Rectangle(double w, double h){
width = w;
height = h;
}
double getarea(){
return width * height;
}
double getperimeter(){
return 2 * (width + height);
}
};
无参构造方法Rectangle()可以直接定义在类中。另一个构造方法Rectangle(double w, double h)用于创建指定width和height值的矩形。getarea()方法返回矩形面积,我们可以用width * height计算得到。getperimeter()方法返回矩形周长,我们可以用2 * (width + height)计算得到。
一个完整的示例程序可以是这样:
#include <iostream>
using namespace std;
class Rectangle{
private:
double width = 1;
double height = 1;
public:
Rectangle(){}
Rectangle(double w, double h){
width = w;
height = h;
}
double getarea(){
return width * height;
}
double getperimeter(){
return 2 * (width + height);
}
};
int main(){
Rectangle rect1;
Rectangle rect2(4,5);
cout << "rect1 area: " << rect1.getarea() << endl;
cout << "rect1 perimeter: " << rect1.getperimeter() << endl;
cout << "rect2 area: " << rect2.getarea() << endl;
cout << "rect2 perimeter: " << rect2.getperimeter() << endl;
return 0;
}
在主函数中,我们创建了两个Rectangle对象rect1和rect2。rect1使用默认构造方法创建,rect2使用另一个构造方法创建。输出面积和周长可以使用getarea()和getperimeter()方法。运行程序,我们可以得到以下结果:
rect1 area: 1
rect1 perimeter: 4
rect2 area: 20
rect2 perimeter: 18
这个程序成功地展示了Rectangle类的基本功能,我们可以用它来创建和操纵矩形对象。
### 回答3:
矩形是一种常见的二维图形,可以使用面向对象的思想来设计一个Rectangle类,以便用于计算矩形的面积和周长。
首先,为了代表矩形的长和宽,我们可以创建两个名为width和height的double类型数据域,它们分别表示矩形的宽和高。width和height的默认值都为1。
接下来,为了创建一个默认矩形的无参构造方法,我们可以编写以下代码:
public Rectangle() {
this.width = 1;
this.height = 1;
}
此构造方法将width和height两个数据域设置为默认值1。
接着,为了创建指定width和height值的矩形的构造方法,我们可以编写以下代码:
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
此构造方法的作用是将width和height两个数据域设置为传入的参数值。
接下来,我们需要实现一个名为getArea()的方法,返回该矩形的面积。面积可以通过长和宽相乘得出,代码如下所示:
public double getArea() {
return this.width * this.height;
}
最后,我们需要实现一个名为getPerimeter()的方法,返回周长。周长可以通过两倍长加两倍宽得出,代码如下所示:
public double getPerimeter() {
return 2 * (this.width + this.height);
}
完整的代码如下所示:
public class Rectangle {
private double width;
private double height;
public Rectangle() {
this.width = 1;
this.height = 1;
}
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getArea() {
return this.width * this.height;
}
public double getPerimeter() {
return 2 * (this.width + this.height);
}
}
阅读全文
相关推荐
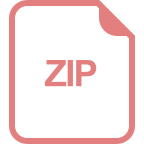
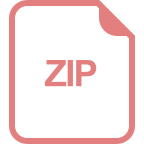
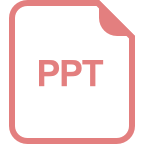










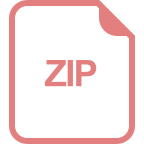
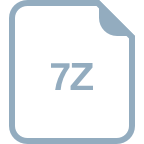
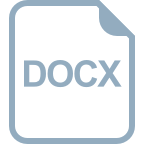