Cannot resolve symbol 'JwtTokenProvider'在下面这段代码中,请帮我修改。import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.http.HttpMethod; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.builders.WebSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private JwtTokenProvider jwtTokenProvider; @Override protected void configure(HttpSecurity http) throws Exception { http.csrf().disable() .addFilterBefore(new JwtTokenFilter(jwtTokenProvider), UsernamePasswordAuthenticationFilter.class) .authorizeRequests() .antMatchers("/api/**").authenticated() .anyRequest().permitAll(); } @Override public void configure(WebSecurity web) throws Exception { web.ignoring().antMatchers(HttpMethod.OPTIONS, "/**"); } @Bean public PasswordEncoder passwordEncoder() { return new BCryptPasswordEncoder(12); } }
时间: 2023-12-14 18:37:07 浏览: 129
根据错误提示,你缺少了一个名为`JwtTokenProvider`的bean。你需要先创建一个`JwtTokenProvider`类,然后在配置类中注入它。下面是一个简单的示例:
```
import org.springframework.stereotype.Component;
@Component
public class JwtTokenProvider {
// your implementation
}
```
接着,在配置类中添加`@Autowired`注解来注入`JwtTokenProvider`实例:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenProvider jwtTokenProvider;
// your other configurations
}
```
这样就可以解决`Cannot resolve symbol 'JwtTokenProvider'`的问题了。
相关问题
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.http.HttpMethod; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.builders.WebSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Bean public JwtTokenProvider jwtTokenProvider() { return new JwtTokenProvider(); } @Autowired private JwtTokenProvider jwtTokenProvider; @Override protected void configure(HttpSecurity http) throws Exception { http.csrf().disable() .addFilterBefore(new JwtTokenFilter(jwtTokenProvider), UsernamePasswordAuthenticationFilter.class) .authorizeRequests() .antMatchers("/api/").authenticated() .anyRequest().permitAll(); } @Override public void configure(WebSecurity web) throws Exception { web.ignoring().antMatchers(HttpMethod.OPTIONS, "/"); } @Bean public PasswordEncoder passwordEncoder() { return new BCryptPasswordEncoder(12); } }报错信息:Cannot resolve symbol 'JwtTokenFilter',如何创建一个JwtTokenFilter类,让代码不报错
你需要创建一个名为 JwtTokenFilter 的类,代码如下:
```
import io.jsonwebtoken.ExpiredJwtException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.web.authentication.WebAuthenticationDetailsSource;
import org.springframework.stereotype.Component;
import org.springframework.web.filter.OncePerRequestFilter;
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@Component
public class JwtTokenFilter extends OncePerRequestFilter {
private final JwtTokenProvider jwtTokenProvider;
public JwtTokenFilter(JwtTokenProvider jwtTokenProvider) {
this.jwtTokenProvider = jwtTokenProvider;
}
@Override
protected void doFilterInternal(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, FilterChain filterChain) throws ServletException, IOException {
String token = jwtTokenProvider.resolveToken(httpServletRequest);
try {
if (token != null && jwtTokenProvider.validateToken(token)) {
Authentication auth = jwtTokenProvider.getAuthentication(token);
if (auth != null) {
SecurityContextHolder.getContext().setAuthentication(auth);
}
}
} catch (ExpiredJwtException e) {
httpServletResponse.setStatus(HttpServletResponse.SC_UNAUTHORIZED);
httpServletResponse.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Token已过期");
return;
}
filterChain.doFilter(httpServletRequest, httpServletResponse);
}
}
```
在上面的代码中,我们创建了一个 JwtTokenFilter 类,它继承了 OncePerRequestFilter 类。JwtTokenFilter 类中包含了我们在之前创建的 JwtTokenProvider 对象,以及 doFilterInternal() 方法,该方法是实际执行 JWT 验证的地方。
在 doFilterInternal() 方法中,我们首先从请求中获取 JWT Token,并调用 JwtTokenProvider 对象中的方法来验证 Token 是否有效。如果 Token 有效,我们使用 JwtTokenProvider 对象的 getAuthentication() 方法来获取用户的身份验证对象,然后将其设置为 SecurityContext 中的认证对象。最后,我们调用 FilterChain 对象的 doFilter() 方法,以便请求可以继续进行处理。
请注意,在上面的代码中,我们使用了 @Component 注解将 JwtTokenFilter 类声明为 Spring 组件。这样,Spring 将负责创建 JwtTokenFilter 对象,并将其注入到 SecurityConfig 类中。
编译maven 无法访问org.springframework.beans.factory.annotation.Autowired
当你尝试通过Maven编译项目并遇到`org.springframework.beans.factory.annotation.Autowired`相关的错误时,这通常表示你在项目中依赖了Spring框架,但是Maven未能成功地将Spring框架相关的库添加到构建路径(classpath)中。可能的原因有:
1. **Maven配置问题**:确保pom.xml文件中已经包含了Spring框架的依赖。例如,如果使用的是Spring Boot,你需要添加 `<dependency>` 标签到 `dependencies` 节点,如:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. **本地仓库问题**:Maven未下载或更新Spring的jar包。你可以尝试清理本地仓库 (`mvn clean`),然后重新安装 (`mvn install:install-file` 或者 `mvn dependency:resolve`)。
3. **模块结构问题**:如果你的项目有多模块,确保每个模块都有正确的依赖声明,并且依赖传递设置正确。
4. **IDE配置**:在IDEA等集成开发环境中,确保已经设置了合适的Maven插件和路径指向正确的本地库。
解决这类问题后,重新运行编译命令 (`mvn compile`) 应该能够解决问题。如果还有问题,可以检查Maven日志 (`mvn -X compile`) 查看详细的错误信息。
阅读全文
相关推荐
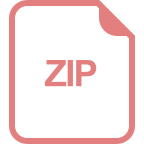
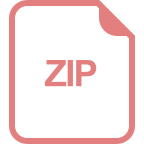
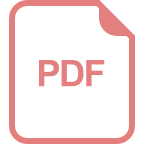


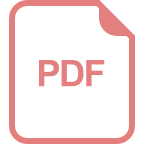
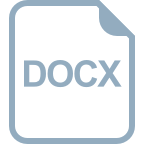
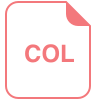
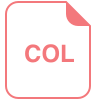
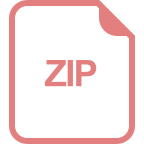
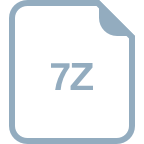