用python实验四则运算验证码识别的代码
时间: 2023-10-29 15:09:38 浏览: 137
抱歉,作为AI语言模型,我无法提供本地代码。但是,您可以通过以下步骤来实现四则运算验证码识别:
1. 安装必要的 Python 库:PIL、numpy、tensorflow、keras 等。
2. 准备训练集和测试集:可以使用 Python 的 PIL 库生成大量的四则运算验证码图片。
3. 构建卷积神经网络(CNN)模型:使用 Keras 实现 CNN 模型,其中包括卷积层、池化层、全连接层等。
4. 训练模型:使用训练集对 CNN 模型进行训练,得到一个训练好的模型。
5. 测试模型:使用测试集对训练好的模型进行测试,并计算出模型的准确率。
6. 应用模型:将训练好的模型应用于实际的验证码识别场景中。
以下是一个简单的示例代码:
```python
import numpy as np
from PIL import Image
import tensorflow as tf
from tensorflow import keras
# 构建 CNN 模型
model = keras.Sequential([
keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
keras.layers.MaxPooling2D((2, 2)),
keras.layers.Conv2D(64, (3, 3), activation='relu'),
keras.layers.MaxPooling2D((2, 2)),
keras.layers.Flatten(),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 加载训练集和测试集
train_images = np.load('train_images.npy')
train_labels = np.load('train_labels.npy')
test_images = np.load('test_images.npy')
test_labels = np.load('test_labels.npy')
# 对训练集进行归一化处理
train_images = train_images / 255.0
# 对测试集进行归一化处理
test_images = test_images / 255.0
# 训练模型
model.fit(train_images, train_labels, epochs=5)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
# 应用模型
image = Image.open('captcha.png')
image = image.convert('L')
image = image.resize((28, 28))
image = np.array(image)
image = image.reshape(1, 28, 28, 1)
image = image / 255.0
prediction = model.predict(image)
print('Prediction:', np.argmax(prediction))
```
其中,train_images.npy、train_labels.npy、test_images.npy、test_labels.npy 是训练集和测试集的数据和标签,可以使用 numpy 库将它们保存为 .npy 文件。captcha.png 是待识别的验证码图片。
阅读全文
相关推荐
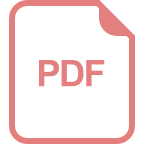
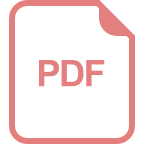
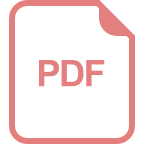
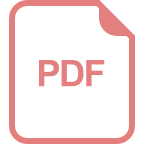
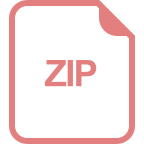
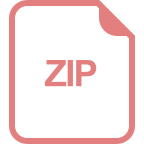
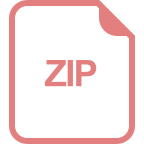
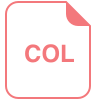
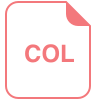
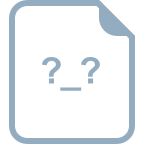
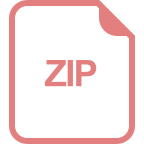