调用ffmpeg g726LE编码器将 pcm音频转为g726 40kbps
时间: 2023-07-05 22:21:06 浏览: 106
实现将 PCM 音频转换为 G.726 LE 编码格式的过程可以分为以下几个步骤:
1. 初始化 FFmpeg 库,并找到 G.726 LE 编码器。
```python
import ffmpeg
# 初始化 FFmpeg 库
ffmpeg.init()
# 查找 G.726 LE 编码器
codec = ffmpeg.avcodec_find_encoder(ffmpeg.AV_CODEC_ID_ADPCM_G726LE)
```
2. 创建 AVCodecContext 对象,并设置编码参数。
```python
# 创建 AVCodecContext 对象
codec_context = ffmpeg.avcodec_alloc_context3(codec)
# 设置编码参数
codec_context.bits_per_coded_sample = 2
codec_context.sample_rate = 8000
codec_context.channels = 1
codec_context.frame_size = 320
codec_context.bit_rate = 40000
```
其中,bits_per_coded_sample 表示每个采样的编码位数,这里是 2;sample_rate 表示采样率,这里是 8000;channels 表示通道数,这里是 1;frame_size 表示编码帧大小,这里是 320;bit_rate 表示比特率,这里是 40000。
3. 打开编码器,并分配编码器数据包和缓冲区。
```python
# 打开编码器
ffmpeg.avcodec_open2(codec_context, codec)
# 分配编码器数据包和缓冲区
pkt = ffmpeg.av_packet_alloc()
frame = ffmpeg.av_frame_alloc()
frame.data[0] = ffmpeg.av_malloc(codec_context.frame_size)
frame.nb_samples = codec_context.frame_size // 2
```
其中,pkt 表示编码器的数据包,frame 表示编码器的帧数据,frame.data[0] 表示帧数据的缓冲区,frame.nb_samples 表示帧数据采样数,这里是 320 // 2 = 160。
4. 读取 PCM 音频数据,并将其转换为 G.726 LE 编码格式。
```python
# 读取 PCM 音频数据
pcm_data = open("audio.pcm", "rb").read()
# 将 PCM 音频数据转换为 G.726 LE 编码格式
while len(pcm_data) >= codec_context.frame_size * 2:
# 将 PCM 数据写入帧数据缓冲区
ffmpeg.swig_opaque(frame.data[0], pcm_data[:codec_context.frame_size * 2])
pcm_data = pcm_data[codec_context.frame_size * 2:]
# 编码帧数据为 G.726 LE 格式
ret = ffmpeg.avcodec_send_frame(codec_context, frame)
while ret >= 0:
ret = ffmpeg.avcodec_receive_packet(codec_context, pkt)
if ret == ffmpeg.AVERROR_EAGAIN or ret == ffmpeg.AVERROR_EOF:
break
elif ret < 0:
raise Exception("Error during encoding")
# 将编码后的数据写入文件
open("audio.g726", "ab").write(pkt.data[:pkt.size])
```
其中,pcm_data 表示待编码的 PCM 音频数据,每次从 PCM 数据中读取 codec_context.frame_size * 2 个字节的数据,将其写入帧数据缓冲区 frame.data[0] 中,并调用 avcodec_send_frame 函数将帧数据送入编码器中编码。编码器编码完成后,通过 avcodec_receive_packet 函数获取编码后的数据包 pkt,将其写入文件中。
5. 释放资源。
```python
# 释放资源
ffmpeg.av_packet_unref(pkt)
ffmpeg.av_frame_free(frame)
ffmpeg.av_freep(frame.data)
ffmpeg.avcodec_close(codec_context)
ffmpeg.avcodec_free_context(codec_context)
```
最后,需要释放编码器数据包、帧数据和编码器上下文等资源。
完整代码如下:
```python
import ffmpeg
# 初始化 FFmpeg 库
ffmpeg.init()
# 查找 G.726 LE 编码器
codec = ffmpeg.avcodec_find_encoder(ffmpeg.AV_CODEC_ID_ADPCM_G726LE)
# 创建 AVCodecContext 对象
codec_context = ffmpeg.avcodec_alloc_context3(codec)
# 设置编码参数
codec_context.bits_per_coded_sample = 2
codec_context.sample_rate = 8000
codec_context.channels = 1
codec_context.frame_size = 320
codec_context.bit_rate = 40000
# 打开编码器
ffmpeg.avcodec_open2(codec_context, codec)
# 分配编码器数据包和缓冲区
pkt = ffmpeg.av_packet_alloc()
frame = ffmpeg.av_frame_alloc()
frame.data[0] = ffmpeg.av_malloc(codec_context.frame_size)
frame.nb_samples = codec_context.frame_size // 2
# 读取 PCM 音频数据
pcm_data = open("audio.pcm", "rb").read()
# 将 PCM 音频数据转换为 G.726 LE 编码格式
while len(pcm_data) >= codec_context.frame_size * 2:
# 将 PCM 数据写入帧数据缓冲区
ffmpeg.swig_opaque(frame.data[0], pcm_data[:codec_context.frame_size * 2])
pcm_data = pcm_data[codec_context.frame_size * 2:]
# 编码帧数据为 G.726 LE 格式
ret = ffmpeg.avcodec_send_frame(codec_context, frame)
while ret >= 0:
ret = ffmpeg.avcodec_receive_packet(codec_context, pkt)
if ret == ffmpeg.AVERROR_EAGAIN or ret == ffmpeg.AVERROR_EOF:
break
elif ret < 0:
raise Exception("Error during encoding")
# 将编码后的数据写入文件
open("audio.g726", "ab").write(pkt.data[:pkt.size])
# 释放资源
ffmpeg.av_packet_unref(pkt)
ffmpeg.av_frame_free(frame)
ffmpeg.av_freep(frame.data)
ffmpeg.avcodec_close(codec_context)
ffmpeg.avcodec_free_context(codec_context)
```
相关推荐
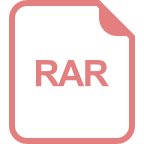
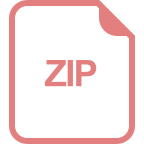














