用C语言写出链地址法处理冲突实现散列表查找的代码
时间: 2023-06-18 12:03:28 浏览: 142
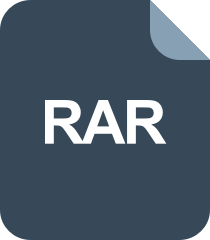
c语言 散列表的设计与实现

下面是使用链地址法处理冲突实现散列表查找的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 10
struct node {
char key[10];
int value;
struct node *next;
};
struct hash_table {
struct node *head;
};
void init_hash_table(struct hash_table *hash_table) {
int i;
for (i = 0; i < MAXSIZE; i++) {
hash_table[i].head = NULL;
}
}
int hash_func(char *key) {
int i, sum = 0;
for (i = 0; i < strlen(key); i++) {
sum += key[i];
}
return sum % MAXSIZE;
}
void insert_node(struct hash_table *hash_table, char *key, int value) {
int index = hash_func(key);
struct node *new_node = (struct node *)malloc(sizeof(struct node));
strcpy(new_node->key, key);
new_node->value = value;
new_node->next = NULL;
if (hash_table[index].head == NULL) {
hash_table[index].head = new_node;
} else {
struct node *p = hash_table[index].head;
while (p->next != NULL) {
p = p->next;
}
p->next = new_node;
}
}
int search_node(struct hash_table *hash_table, char *key) {
int index = hash_func(key);
struct node *p = hash_table[index].head;
while (p != NULL) {
if (strcmp(p->key, key) == 0) {
return p->value;
}
p = p->next;
}
return -1;
}
int main() {
struct hash_table hash_table[MAXSIZE];
init_hash_table(hash_table);
insert_node(hash_table, "apple", 10);
insert_node(hash_table, "banana", 20);
insert_node(hash_table, "cat", 30);
printf("The value of apple is %d\n", search_node(hash_table, "apple"));
printf("The value of banana is %d\n", search_node(hash_table, "banana"));
printf("The value of cat is %d\n", search_node(hash_table, "cat"));
printf("The value of dog is %d\n", search_node(hash_table, "dog"));
return 0;
}
```
这里使用了一个结构体数组来表示散列表,每个数组元素包含一个链表的头指针。`init_hash_table` 函数用于初始化散列表,将每个头指针都设置为 `NULL`。`hash_func` 函数用于计算关键字对应的散列值,这里采用了简单的求和方法。`insert_node` 函数用于向散列表中插入一个节点,如果该位置的链表为空,则直接将该节点作为链表的头节点;否则将该节点插入到链表的末尾。`search_node` 函数用于查找散列表中是否有对应的关键字,如果有,则返回其对应的值,否则返回 `-1`。
在 `main` 函数中,我们向散列表中插入了三个节点,然后分别查找了三个关键字的值,以及一个不存在的关键字的值,最后将结果输出。
阅读全文
相关推荐
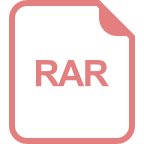

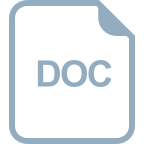



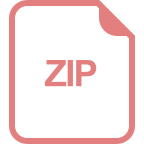
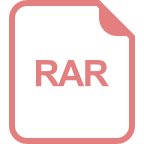
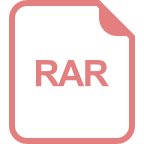
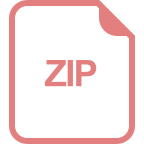
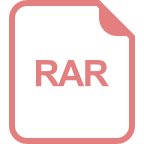
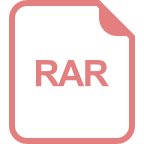
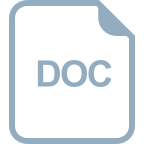
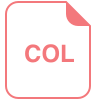


