android底部导航栏navigation,BottomNavigationView,给出各部分具体代码,最好以一个demo的形式给出完整的代码
时间: 2024-02-13 13:05:17 浏览: 59
好的,以下是一个简单的 Android 应用程序,其中包括一个底部导航栏(BottomNavigationView)。
首先,我们需要在 `build.gradle` 文件中添加 `implementation 'com.google.android.material:material:1.2.1'`,这将为我们提供 Material Design 库,其中包括 BottomNavigationView。
接下来,我们需要在 `activity_main.xml` 文件中添加一个 BottomNavigationView:
```xml
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/bottom_navigation"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
app:menu="@menu/bottom_navigation_menu" />
```
`menu` 属性是一个指向我们的菜单资源文件的引用,我们需要在 `res` 文件夹下创建一个名为 `menu` 的文件夹,并在其中创建一个名为 `bottom_navigation_menu.xml` 的文件,它应该如下所示:
```xml
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/navigation_home"
android:icon="@drawable/ic_home"
android:title="@string/title_home" />
<item
android:id="@+id/navigation_dashboard"
android:icon="@drawable/ic_dashboard"
android:title="@string/title_dashboard" />
<item
android:id="@+id/navigation_notifications"
android:icon="@drawable/ic_notifications"
android:title="@string/title_notifications" />
</menu>
```
现在,我们需要在我们的 `MainActivity` 中使用 BottomNavigationView。以下是 MainActivity 的完整代码:
```java
public class MainActivity extends AppCompatActivity {
private BottomNavigationView bottomNavigationView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bottomNavigationView = findViewById(R.id.bottom_navigation);
bottomNavigationView.setOnNavigationItemSelectedListener(navListener);
getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container,
new HomeFragment()).commit();
}
private BottomNavigationView.OnNavigationItemSelectedListener navListener =
new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
Fragment selectedFragment = null;
switch (item.getItemId()) {
case R.id.navigation_home:
selectedFragment = new HomeFragment();
break;
case R.id.navigation_dashboard:
selectedFragment = new DashboardFragment();
break;
case R.id.navigation_notifications:
selectedFragment = new NotificationsFragment();
break;
}
getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container,
selectedFragment).commit();
return true;
}
};
}
```
我们在 `onCreate` 方法中获取 BottomNavigationView,并为其设置一个 `OnNavigationItemSelectedListener`,以便在用户选择某个导航项时切换 Fragment。我们还在 `onCreate` 方法中添加了一个名为 `HomeFragment` 的 Fragment,这是我们默认显示的 Fragment。
现在,我们需要为每个导航项创建一个 Fragment。以下是我们的三个 Fragment:
```java
public class HomeFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_home, container, false);
}
}
public class DashboardFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_dashboard, container, false);
}
}
public class NotificationsFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_notifications, container, false);
}
}
```
最后,我们需要创建我们的三个 Fragment 布局文件。这些布局文件可以包含任何你想要的内容,我将使用一个简单的 TextView 演示:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Home Fragment" />
</RelativeLayout>
```
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Dashboard Fragment" />
</RelativeLayout>
```
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Notifications Fragment" />
</RelativeLayout>
```
这样,我们就完成了一个简单的 Android 应用程序,其中包括一个底部导航栏。
阅读全文
相关推荐

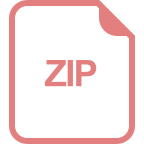
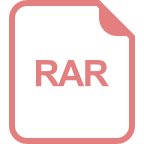
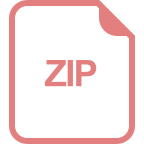
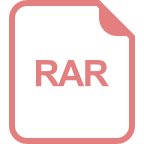
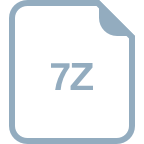
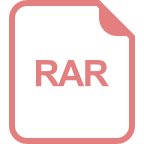
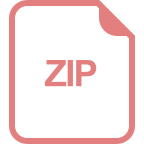
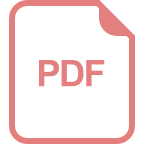
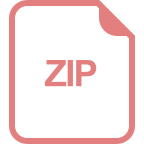
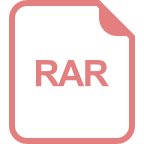
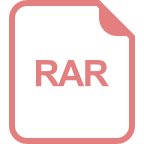
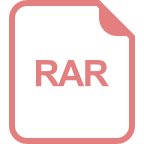
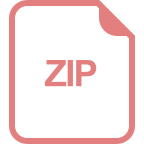
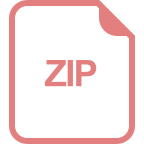
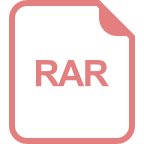