c语言编写一段代码实现以下功能:在 steg.c 中,完成 struct PPM 的声明,它保存从 PPM 文件中读取的图像。这将需要包括来自 PPM 头文件的信息(宽度、高度、最大值),以及指向动态分配的包含像素数据的数组的指针。您的程序应该能够处理任意行数和列数的 PPM 文件。
时间: 2024-02-10 08:10:51 浏览: 36
以下是实现上述功能的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct PPM {
int width;
int height;
int max;
unsigned char* pixels;
};
// 读取 PPM 文件
struct PPM* getPPM(FILE* f) {
char magic[3];
int width, height, max;
fscanf(f, "%2s\n%d %d\n%d\n", magic, &width, &height, &max);
if (magic[0] != 'P' || magic[1] != '6') {
fprintf(stderr, "Error: not a PPM file\n");
return NULL;
}
struct PPM* img = (struct PPM*) malloc(sizeof(struct PPM));
if (img == NULL) {
fprintf(stderr, "Error: failed to allocate memory\n");
return NULL;
}
img->width = width;
img->height = height;
img->max = max;
img->pixels = (unsigned char*) malloc(width * height * 3 * sizeof(unsigned char));
if (img->pixels == NULL) {
fprintf(stderr, "Error: failed to allocate memory\n");
free(img);
return NULL;
}
fread(img->pixels, sizeof(unsigned char), width * height * 3, f);
return img;
}
int main() {
FILE* f = fopen("test.ppm", "r");
struct PPM* img = getPPM(f);
printf("Width: %d\n", img->width);
printf("Height: %d\n", img->height);
printf("Max value: %d\n", img->max);
free(img->pixels);
free(img);
fclose(f);
return 0;
}
```
该代码将从名为 test.ppm 的 PPM 文件中读取图像,并输出其宽度、高度和最大值。注意,此代码仅处理 P6 类型的 PPM 文件,不会处理注释。
相关推荐
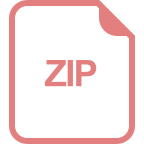
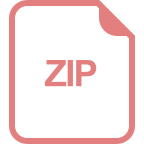


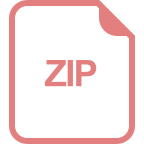
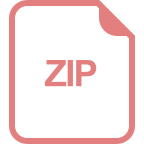
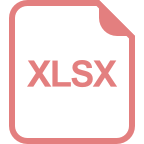
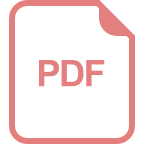
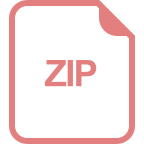
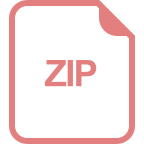