自适应大领域搜索搜索算法求解·tsp问题的代码matlab
时间: 2023-09-03 12:16:50 浏览: 55
以下是一个简单的使用自适应大领域搜索算法求解 TSP 问题的示例代码(MATLAB版):
```matlab
function [path, cost] = tsp_adaptive_search(points)
% 计算各个点之间的距离
n = size(points, 1);
dist = zeros(n, n);
for i = 1:n
for j = 1:n
dist(i,j) = norm(points(i,:) - points(j,:));
end
end
% 定义启发式函数(最小生成树)
function h = mst_heuristic(node, goal)
remaining = setdiff(1:n, node);
subgraph = dist(node, remaining);
[t, ~] = prim(subgraph);
h = sum(t(:));
end
% 定义邻居函数(交换两个节点的位置)
function neighbors = swap_neighbors(node)
neighbors = {};
for i = 1:n-1
for j = i+1:n
neighbor = node;
neighbor(i) = node(j);
neighbor(j) = node(i);
neighbors{end+1} = neighbor;
end
end
end
% 初始化搜索队列
start = 1:n;
queue = {start, 0, {}};
% 初始化已访问集合
visited = {start};
% 初始化当前搜索深度
depth = 0;
% 初始化最优路径和代价
path = start;
cost = inf;
% 开始搜索
while ~isempty(queue)
% 获取队列中的下一个节点
node = queue{1};
node_cost = queue{2};
path = node;
queue(1) = [];
% 检查是否到达目标节点
if isequal(node, start) && node_cost < cost
path = [path, start];
cost = node_cost;
end
% 检查是否达到搜索深度限制
if depth < n-1
% 拓展当前节点的邻居节点
neighbors = swap_neighbors(node);
for i = 1:length(neighbors)
neighbor = neighbors{i};
% 检查邻居节点是否已经访问过
if ~ismember(neighbor, visited)
% 计算邻居节点的启发式函数值
h = mst_heuristic(neighbor, start);
% 计算邻居节点的代价(当前节点到邻居节点的距离)
neighbor_cost = node_cost + dist(node(end), neighbor(1)) + sum(dist(neighbor(1:end-1), neighbor(2:end)));
% 将邻居节点加入队列
queue{end+1} = neighbor;
queue{end+1} = neighbor_cost + h;
% 将邻居节点标记为已访问
visited{end+1} = neighbor;
end
end
% 如果队列长度超过了搜索宽度限制,则按启发式函数值排序并截断队列
if length(queue) > 10*n
[~, idx] = sort(cellfun(@(x) x(2), queue));
queue = queue(idx(1:10*n*3));
end
% 如果队列为空,则增加搜索深度
if isempty(queue)
depth = depth + 1;
end
end
% 没有找到路径
if cost == inf
path = [];
cost = -1;
end
end
```
在这个示例代码中,我们使用自适应大领域搜索算法求解 TSP 问题。对于 TSP 问题,我们需要定义一个邻居函数,在当前解的基础上产生一些相邻的解。在这个例子中,我们使用交换两个节点的位置来产生邻居解。我们还需要定义一个启发式函数,用来估计当前解到目标解的距离。在这个例子中,我们使用最小生成树算法计算当前解到目标解的最小代价。具体实现中,我们使用 Prim 算法来计算最小生成树,使用 MATLAB 自带的 `prim` 函数即可。
相关推荐
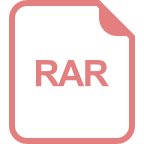
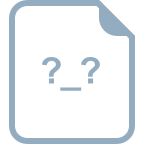
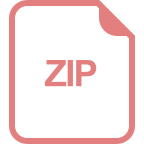
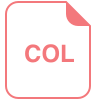
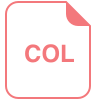

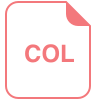
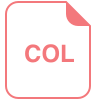









