public class StudentDemo { public static void main(String[] args) { Scanner in = new Scanner(System.in); // 判断类型并循环输入 String stuNo, name, str; double english, math, Java, testScore = 1.0; while (in.hasNext()) { String line = in.nextLine(); String[] fields = line.split(" "); //字符串 line 按照空格分隔符进行拆分,拆分后的结果存储在一个名为 fields 的字符串数组中 stuNo = fields[0]; //取 fields 数组的第一个元素,即该语句将 fields 数组的第一个元素的值赋给了变量 stuNo name = fields[1]; english = Double.parseDouble(fields[2]); math = Double.parseDouble(fields[3]); Java = Double.parseDouble(fields[4]); if (fields.length == 5) { // 学生PT Student s1 = new StudentPT(stuNo, name, english, math, Java, testScore); System.out.println(s1.toString()); } else if (fields[5].equals("SystemFramework")) { // 学生SJ Student s2 = new StudentSJ(stuNo, name, english, math, Java, testScore); System.out.println(s2.toString() + " task:SystemFramework"); double sum2 = s2.sum(); } else if (fields[5].equals("GroupLeader")) { // 学生ZZ Student s3 = new StudentZZ(stuNo, name, english, math, Java, testScore); System.out.println(s3.toString() + " duty:GroupLeader"); double sum3 = s3.sum(); } else { break; } } //比较大小 double max = Math.max(sum2,sum3); if(max == sum2){ System.out.println("Lisi's total score is more than Wangwu"); } } } 在sum部分有什么错误
时间: 2023-12-10 08:03:05 浏览: 33
在代码中有两个地方使用了变量 `sum2` 和 `sum3`,但是这两个变量是在 if/else 分支中定义的,而在这个代码中,它们只能在对应的 if/else 分支中使用。因此,在比较大小的地方使用 `sum2` 和 `sum3` 会导致编译错误。要解决这个问题,可以将 `sum2` 和 `sum3` 的定义移动到 while 循环外部,这样它们就可以在整个 main 函数中使用了。例如:
```
public class StudentDemo {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
// 判断类型并循环输入
String stuNo, name, str;
double english, math, Java, testScore = 1.0;
double sum2 = 0, sum3 = 0; // 将 sum2 和 sum3 的定义移动到循环外部
while (in.hasNext()) {
String line = in.nextLine();
String[] fields = line.split(" ");
stuNo = fields[0];
name = fields[1];
english = Double.parseDouble(fields[2]);
math = Double.parseDouble(fields[3]);
Java = Double.parseDouble(fields[4]);
if (fields.length == 5) {
// 学生PT
Student s1 = new StudentPT(stuNo, name, english, math, Java, testScore);
System.out.println(s1.toString());
} else if (fields[5].equals("SystemFramework")) {
// 学生SJ
Student s2 = new StudentSJ(stuNo, name, english, math, Java, testScore);
System.out.println(s2.toString() + " task:SystemFramework");
sum2 = s2.sum(); // 将 sum2 和 sum3 的赋值移到对应的 if/else 分支中
} else if (fields[5].equals("GroupLeader")) {
// 学生ZZ
Student s3 = new StudentZZ(stuNo, name, english, math, Java, testScore);
System.out.println(s3.toString() + " duty:GroupLeader");
sum3 = s3.sum();
} else {
break;
}
}
//比较大小
double max = Math.max(sum2, sum3);
if (max == sum2) {
System.out.println("Lisi's total score is more than Wangwu");
}
}
}
```
相关推荐
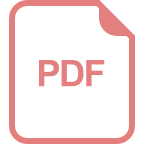
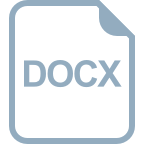
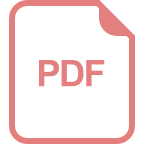















