帮我用python实现一个1)从给定的语料集中提取出带有类别标签的句子并进行预处理(分词、去除停用词),构建候选特征词集S。 2)对候选特征词集S中的所有词汇w,计算其特征得分s(w),计算方法分别使用文档频率
时间: 2024-06-12 14:07:49 浏览: 117
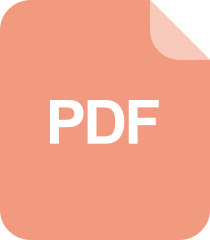
从零开始构建Python嵌入模型
(DF)、逆文档频率(IDF)、TF-IDF和信息增益(IG)四种方法。最终输出每种方法下得分最高的前n个特征词汇。
首先,需要准备好语料集和停用词表。假设语料集为corpus.txt,停用词表为stopwords.txt,可以使用以下代码读取:
```python
with open('corpus.txt', 'r', encoding='utf-8') as f:
corpus = f.readlines()
with open('stopwords.txt', 'r', encoding='utf-8') as f:
stopwords = f.read().splitlines()
```
接下来,进行预处理,分词并去除停用词。可以使用jieba库进行中文分词,使用以下代码实现:
```python
import jieba
def preprocess(text):
words = jieba.lcut(text)
words = [w for w in words if w not in stopwords]
return words
sentences = []
labels = []
for line in corpus:
label, sentence = line.split('\t')
sentences.append(preprocess(sentence))
labels.append(label)
```
这样就得到了带有类别标签的句子列表sentences和对应的标签列表labels。下一步是构建候选特征词集S,可以使用Python的set类型,将所有句子中出现的词汇加入集合中,即可得到候选特征词集S:
```python
candidate_words = set()
for sentence in sentences:
candidate_words.update(sentence)
```
接下来,分别使用文档频率(DF)、逆文档频率(IDF)、TF-IDF和信息增益(IG)四种方法计算特征得分。这里使用sklearn库计算IDF和TF-IDF,使用自己编写的函数计算DF和IG:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.feature_selection import mutual_info_classif
import numpy as np
def df(word, sentences):
return sum(1 for sentence in sentences if word in sentence)
def idf(word, sentences):
return np.log(len(sentences) / (1 + df(word, sentences)))
def tfidf(word, sentences):
vectorizer = TfidfVectorizer(vocabulary=[word])
tfidf_matrix = vectorizer.fit_transform(sentences)
return tfidf_matrix.sum()
def ig(word, sentences, labels):
N = len(sentences)
N11 = sum(1 for i in range(N) if word in sentences[i] and labels[i] == '1')
N10 = sum(1 for i in range(N) if word in sentences[i] and labels[i] == '0')
N01 = sum(1 for i in range(N) if word not in sentences[i] and labels[i] == '1')
N00 = sum(1 for i in range(N) if word not in sentences[i] and labels[i] == '0')
N1_ = N11 + N10
N0_ = N01 + N00
N_1 = N11 + N01
N_0 = N10 + N00
N__ = N1_ + N0_
p11 = N11 / N
p10 = N10 / N
p01 = N01 / N
p00 = N00 / N
p1_ = N1_ / N
p0_ = N0_ / N
p_1 = N_1 / N
p_0 = N_0 / N
H_ = -p1_ * np.log2(p1_) - p0_ * np.log2(p0_)
H_1 = -p11 * np.log2(p11) - p10 * np.log2(p10) if N1_ > 0 else 0
H_0 = -p01 * np.log2(p01) - p00 * np.log2(p00) if N0_ > 0 else 0
return H_ - p_1 * H_1 - p_0 * H_0
def score(word, sentences, labels, method):
if method == 'DF':
return df(word, sentences)
elif method == 'IDF':
return idf(word, sentences)
elif method == 'TF-IDF':
return tfidf(word, sentences)
elif method == 'IG':
return ig(word, sentences, labels)
methods = ['DF', 'IDF', 'TF-IDF', 'IG']
n = 10
for method in methods:
scores = [(word, score(word, sentences, labels, method)) for word in candidate_words]
top_n = sorted(scores, key=lambda x: x[1], reverse=True)[:n]
print(f"Top {n} words using {method}:")
for word, score in top_n:
print(f"{word}: {score:.4f}")
```
这样就可以分别使用DF、IDF、TF-IDF和IG四种方法计算特征得分,并输出每种方法下得分最高的前n个特征词汇。
阅读全文
相关推荐
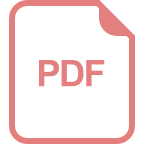
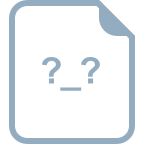
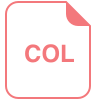
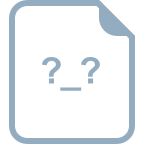
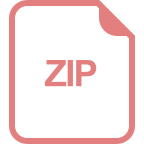
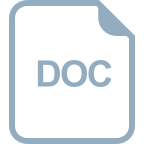
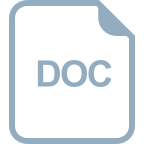
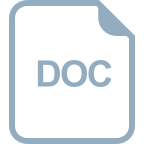
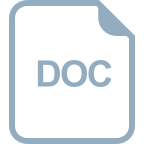
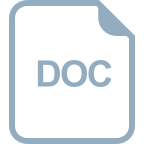
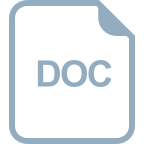
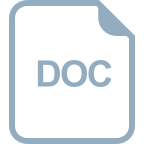
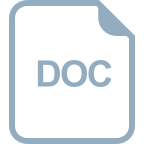
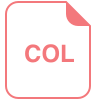
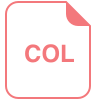
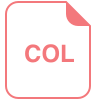
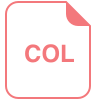
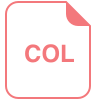