图片拖拽排序vue代码
时间: 2023-08-31 17:29:05 浏览: 82
### 回答1:
以下是一个简单的 Vue.js 实现图片拖拽排序的代码示例:
HTML 部分:
```
<template>
<div>
<ul>
<li v-for="(item, index) in items" :key="index" @dragstart="dragStart(index)" @dragend="dragEnd" draggable="true">
<img :src="item" />
</li>
</ul>
</div>
</template>
```
JS 部分:
```
<script>
export default {
data() {
return {
items: [
'https://via.placeholder.com/100x100',
'https://via.placeholder.com/100x100',
'https://via.placeholder.com/100x100'
],
currentIndex: null
}
},
methods: {
dragStart(index) {
this.currentIndex = index
},
dragEnd() {
this.currentIndex = null
},
drop(toIndex) {
const currentItem = this.items[this.currentIndex]
this.items.splice(this.currentIndex, 1)
this.items.splice(toIndex, 0, currentItem)
}
}
}
</script>
```
该代码实现了一个图片列表,每个图片都可以通过拖拽进行排序。在 `dragstart` 事件中记录当前图片的索引,在 `dragend` 事件中清空当前图片的索引,在 `drop` 事件中改变图片列表的顺序。
该代码仅提供一个示例,在实际开发中可能需要根据需求进行修改和完善。
### 回答2:
图片拖拽排序是一种常见的前端交互效果,可以让用户自由拖动图片进行排序。下面是一个用Vue实现的图片拖拽排序的代码示例:
```html
<template>
<div>
<h2>图片拖拽排序</h2>
<div class="image-list">
<div
class="image-item"
v-for="(image, index) in images"
:key="image.id"
:style="{ order: image.order }"
draggable="true"
@dragstart="onDragStart(index)"
@dragover="onDragOver(index)"
@drop="onDrop(index)"
>
<img :src="image.url" alt="image" />
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
images: [
{ id: 1, url: 'image1.jpg', order: 1 },
{ id: 2, url: 'image2.jpg', order: 2 },
{ id: 3, url: 'image3.jpg', order: 3 },
{ id: 4, url: 'image4.jpg', order: 4 },
],
draggingIndex: null,
dropIndex: null,
};
},
methods: {
onDragStart(index) {
this.draggingIndex = index;
},
onDragOver(index) {
event.preventDefault();
this.dropIndex = index;
},
onDrop() {
const draggingImage = this.images[this.draggingIndex];
const dropImage = this.images[this.dropIndex];
this.images.splice(this.draggingIndex, 1);
this.images.splice(this.dropIndex, 0, draggingImage);
this.draggingIndex = null;
this.dropIndex = null;
},
},
};
</script>
<style scoped>
.image-list {
display: flex;
flex-wrap: wrap;
}
.image-item {
width: 200px;
height: 200px;
margin: 10px;
}
img {
width: 100%;
height: 100%;
object-fit: cover;
}
</style>
```
上述代码中,images数组存储了要展示的图片信息,每个图片对象包含id、url和order属性。在组件的模板中,通过v-for指令遍历images数组并渲染图片元素。每个图片元素设置draggable="true",并监听相关的drag事件。onDragStart方法记录拖拽开始时的图片索引,onDragOver方法阻止默认行为并记录拖拽结束时的目标位置索引,onDrop方法根据目标位置索引重新排序images数组,并重置拖拽开始和结束的索引。
最终,用户可以通过拖拽图片实现排序,拖拽过程中会展示视觉上的交互效果。
相关推荐
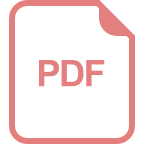
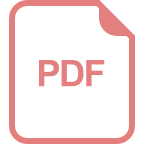
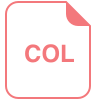













