python实现电子词典
时间: 2023-06-29 07:07:57 浏览: 53
实现一个简单的电子词典,可以使用 Python 内置的字典数据类型和文件操作。
首先,我们需要一个包含单词和对应释义的文本文件,例如:
```
apple: a round fruit with red or green skin and a white inside
banana: a long curved fruit with a yellow skin
orange: a round citrus fruit with a tough bright reddish-yellow rind
```
我们可以使用 `open` 函数打开这个文件,并将它读取到一个 Python 字典中:
```python
with open('dictionary.txt') as f:
lines = f.readlines()
dictionary = {}
for line in lines:
word, definition = line.strip().split(': ')
dictionary[word] = definition
```
现在我们就可以根据用户输入的单词来查询它的释义了:
```python
while True:
word = input('Enter a word (q to quit): ')
if word == 'q':
break
if word in dictionary:
print(dictionary[word])
else:
print('Sorry, that word is not in the dictionary.')
```
这个程序可以不断地接受用户输入,直到用户输入 `q` 退出。如果用户输入的单词在字典中存在,就输出对应的释义;否则输出提示信息。
完整的代码如下:
```python
with open('dictionary.txt') as f:
lines = f.readlines()
dictionary = {}
for line in lines:
word, definition = line.strip().split(': ')
dictionary[word] = definition
while True:
word = input('Enter a word (q to quit): ')
if word == 'q':
break
if word in dictionary:
print(dictionary[word])
else:
print('Sorry, that word is not in the dictionary.')
```
注意,这个电子词典只是一个简单的示例,实际应用中可能需要更复杂的功能和更大的词库。
相关推荐
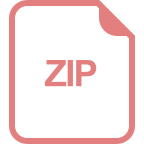









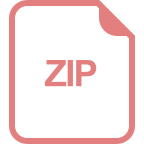
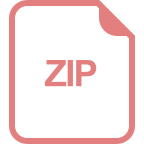
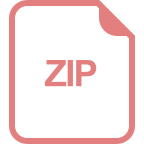
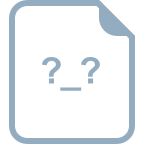
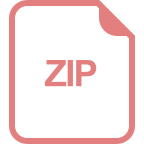