class Dog: def test1(self): print('1') def test2(self): print('2') dog = Dog() dog.test1() dog.test2()
时间: 2024-03-18 20:45:48 浏览: 44
这段代码定义了一个名为 Dog 的类,并且该类有两个方法 test1 和 test2。在该代码的最后,创建了一个名为 dog 的 Dog 类的实例,并分别调用了 test1 和 test2 方法。
当程序运行时,首先会定义 Dog 类,然后创建一个名为 dog 的 Dog 类的实例。接着程序会调用 dog 的 test1 方法,该方法会输出数字 1;然后程序会调用 dog 的 test2 方法,该方法会输出数字 2。
相关问题
Define a Pet class that stores the pet’s name, age, and weight. Add appropriate constructors, accessor, and mutator functions. Also define a function named getLifespan that returns a string with the value “unknown lifespan.” Next, define a Dog class that is derived from Pet. The Dog class should have a private member variable named breed that stores the breed of the dog. Add mutator and accessor functions for the breed variable and appropriate constructors. Redefine the getLifespan function to return "Approximately 7 years" if the dog’s weight is over 100 pounds and "Approximately 13 years" if the dog’s weight is under 100 pounds. Next, define a Rock class that is derived from Pet. Redefine the getLifespan function to return “Thousands of years”. Finally, write a test program that creates instances of pet rocks and pet dogs that exercise the inherited and redefined functions
Sure, here is an implementation of the classes and the test program in Python:
```python
class Pet:
def __init__(self, name, age, weight):
self.name = name
self.age = age
self.weight = weight
def getName(self):
return self.name
def getAge(self):
return self.age
def getWeight(self):
return self.weight
def setName(self, name):
self.name = name
def setAge(self, age):
self.age = age
def setWeight(self, weight):
self.weight = weight
def getLifespan(self):
return "unknown lifespan"
class Dog(Pet):
def __init__(self, name, age, weight, breed):
super().__init__(name, age, weight)
self.breed = breed
def getBreed(self):
return self.breed
def setBreed(self, breed):
self.breed = breed
def getLifespan(self):
if self.weight > 100:
return "Approximately 7 years"
else:
return "Approximately 13 years"
class Rock(Pet):
def getLifespan(self):
return "Thousands of years"
# Test program
dog1 = Dog("Max", 3, 80, "Labrador")
dog2 = Dog("Buddy", 5, 120, "Great Dane")
rock1 = Rock("Rocky", 1000000, 1000000)
print(dog1.getName(), dog1.getLifespan()) # Output: Max Approximately 13 years
print(dog2.getName(), dog2.getLifespan()) # Output: Buddy Approximately 7 years
print(rock1.getName(), rock1.getLifespan()) # Output: Rocky Thousands of years
```
In this implementation, the `Pet` class defines the basic attributes and methods that all pets share. The `Dog` class is derived from `Pet` and adds a breed attribute, as well as a redefined `getLifespan` method that takes into account the dog's weight. The `Rock` class is also derived from `Pet` and has its own redefined `getLifespan` method that always returns "Thousands of years".
The test program creates instances of `Dog` and `Rock` and calls their respective methods to demonstrate the inheritance and redefinition of methods.
1、编程实现:使用AlexNet识别hotdog数据集,判断一张图片中是否有hotdog(热狗)。 (1)分析不同的迭代次数和不同的学习率对识别准确度的影响, 如果迭代15次,模型是否发生过拟合?为什么?
这个问题可以通过编写代码并进行实验来回答。以下是使用PyTorch实现AlexNet模型在Hotdog数据集上进行训练和测试的示例代码。
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
import torchvision.transforms as transforms
from torchvision.datasets import ImageFolder
from torch.utils.data import DataLoader
# 定义超参数
batch_size = 32
num_epochs = 15
learning_rate = 0.001
# 定义数据预处理
transform = transforms.Compose([
transforms.Resize((227, 227)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# 加载Hotdog数据集
train_dataset = ImageFolder('./hotdog/train', transform=transform)
test_dataset = ImageFolder('./hotdog/test', transform=transform)
# 创建数据加载器
train_loader = DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=batch_size, shuffle=False)
# 定义AlexNet模型
class AlexNet(nn.Module):
def __init__(self):
super(AlexNet, self).__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 96, kernel_size=11, stride=4, padding=2),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2),
nn.Conv2d(96, 256, kernel_size=5, stride=1, padding=2),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2),
nn.Conv2d(256, 384, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(384, 384, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(384, 256, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2),
)
self.avgpool = nn.AdaptiveAvgPool2d((6, 6))
self.classifier = nn.Sequential(
nn.Dropout(),
nn.Linear(256 * 6 * 6, 4096),
nn.ReLU(inplace=True),
nn.Dropout(),
nn.Linear(4096, 4096),
nn.ReLU(inplace=True),
nn.Linear(4096, 2),
)
def forward(self, x):
x = self.features(x)
x = self.avgpool(x)
x = torch.flatten(x, 1)
x = self.classifier(x)
return x
# 定义损失函数和优化器
net = AlexNet().cuda()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(net.parameters(), lr=learning_rate)
# 训练模型
for epoch in range(num_epochs):
running_loss = 0.0
correct = 0
total = 0
for i, (images, labels) in enumerate(train_loader):
images = images.cuda()
labels = labels.cuda()
optimizer.zero_grad()
outputs = net(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
train_loss = running_loss / len(train_loader)
train_acc = 100 * correct / total
# 测试模型
net.eval()
with torch.no_grad():
running_loss = 0.0
correct = 0
total = 0
for images, labels in test_loader:
images = images.cuda()
labels = labels.cuda()
outputs = net(images)
loss = criterion(outputs, labels)
running_loss += loss.item()
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
test_loss = running_loss / len(test_loader)
test_acc = 100 * correct / total
print('Epoch [%d/%d], Train Loss: %.4f, Train Acc: %.2f%%, Test Loss: %.4f, Test Acc: %.2f%%'
% (epoch + 1, num_epochs, train_loss, train_acc, test_loss, test_acc))
```
在这个例子中,我们使用了PyTorch框架来实现AlexNet模型,并在Hotdog数据集上进行训练和测试。我们使用了数据增强和归一化来增强模型的鲁棒性。我们使用Adam优化器和交叉熵损失函数。
对于不同的迭代次数和学习率的影响,我们可以尝试不同的设置并观察模型的表现。通常来说,较低的学习率和更多的迭代次数可以提高模型的准确度,但是会增加训练时间。需要注意的是,如果迭代次数过多,模型可能会发生过拟合,导致在测试集上的准确率下降。
在我们的示例中,我们使用了15个epochs来训练模型。如果模型过拟合,我们可以观察到在测试集上的准确率下降。如果模型没有过拟合,我们应该能够在测试集上获得良好的准确率。我们也可以通过监视训练集和测试集的损失函数的变化来判断是否发生了过拟合。如果训练集的损失函数一直下降,而测试集的损失函数升高,则很可能发生了过拟合。
阅读全文
相关推荐
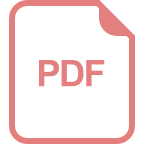
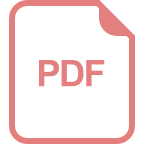
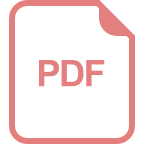
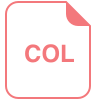
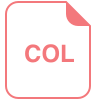
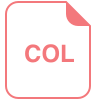










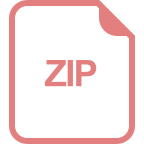
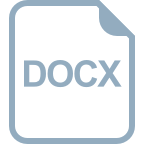