写一个java考试系统的程序,系统应该能够管理不同的考试科目和试题。 学生应该能够注册、登录和参加考试。 考试应该有一定的时间限制,超时后自动提交。 学生应该能够查看自己的考试成绩和历史记录。 教师应该能够添加、修改和删除考试科目和试题,并查看学生的成绩和历史记录。
时间: 2024-03-10 14:51:28 浏览: 16
以下是一个简单的Java考试系统程序示例,包含了基本的考试管理和用户管理功能:
```java
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class ExamSystem {
private Map<String, ExamSubject> subjects; // 考试科目
private Map<String, Student> students; // 学生
private Map<String, Teacher> teachers; // 教师
private Scanner scanner;
public ExamSystem() {
subjects = new HashMap<>();
students = new HashMap<>();
teachers = new HashMap<>();
scanner = new Scanner(System.in);
}
// 添加考试科目
public void addSubject() {
System.out.print("请输入科目名称:");
String name = scanner.nextLine();
System.out.print("请输入考试时间(yyyy-MM-dd HH:mm:ss):");
String timeStr = scanner.nextLine();
Date time = DateUtil.parse(timeStr);
System.out.print("请输入考试时长(分钟):");
int duration = scanner.nextInt();
System.out.print("请输入总分数:");
int totalScore = scanner.nextInt();
scanner.nextLine();
ExamSubject subject = new ExamSubject(name, time, duration, totalScore);
subjects.put(name, subject);
System.out.println("添加科目成功!");
}
// 添加试题
public void addQuestion() {
System.out.print("请输入科目名称:");
String name = scanner.nextLine();
ExamSubject subject = subjects.get(name);
if (subject == null) {
System.out.println("科目不存在!");
return;
}
System.out.print("请输入题目类型(1:单选题,2:多选题,3:判断题):");
int type = scanner.nextInt();
scanner.nextLine();
System.out.print("请输入题目内容:");
String content = scanner.nextLine();
System.out.print("请输入选项个数:");
int optionCount = scanner.nextInt();
scanner.nextLine();
String[] options = new String[optionCount];
for (int i = 0; i < optionCount; i++) {
System.out.print("请输入选项" + (i + 1) + ":");
options[i] = scanner.nextLine();
}
System.out.print("请输入答案(单选题和判断题只需输入一个答案,多选题需要用逗号分隔多个答案):");
String answer = scanner.nextLine();
System.out.print("请输入分值:");
int score = scanner.nextInt();
scanner.nextLine();
Question question = new Question(type, content, options, answer, score);
subject.addQuestion(question);
System.out.println("添加试题成功!");
}
// 注册学生
public void registerStudent() {
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
System.out.print("请输入邮箱:");
String email = scanner.nextLine();
System.out.print("请输入真实姓名:");
String realName = scanner.nextLine();
System.out.print("请输入班级:");
String className = scanner.nextLine();
Student student = new Student(username, password, email, realName, className);
students.put(username, student);
System.out.println("注册成功!");
}
// 注册教师
public void registerTeacher() {
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
System.out.print("请输入邮箱:");
String email = scanner.nextLine();
System.out.print("请输入真实姓名:");
String realName = scanner.nextLine();
Teacher teacher = new Teacher(username, password, email, realName);
teachers.put(username, teacher);
System.out.println("注册成功!");
}
// 学生登录
public void studentLogin() {
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
Student student = students.get(username);
if (student == null || !student.getPassword().equals(password)) {
System.out.println("用户名或密码错误!");
return;
}
studentMenu(student);
}
// 教师登录
public void teacherLogin() {
System.out.print("请输入用户名:");
String username = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
Teacher teacher = teachers.get(username);
if (teacher == null || !teacher.getPassword().equals(password)) {
System.out.println("用户名或密码错误!");
return;
}
teacherMenu(teacher);
}
// 学生菜单
public void studentMenu(Student student) {
while (true) {
System.out.println("欢迎," + student.getRealName() + "!");
System.out.println("1. 参加考试");
System.out.println("2. 查看成绩");
System.out.println("3. 查看历史记录");
System.out.println("4. 退出登录");
System.out.print("请选择操作:");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
takeExam(student);
break;
case 2:
viewScore(student);
break;
case 3:
viewHistory(student);
break;
case 4:
return;
default:
System.out.println("无效的选择!");
break;
}
}
}
// 教师菜单
public void teacherMenu(Teacher teacher) {
while (true) {
System.out.println("欢迎," + teacher.getRealName() + "!");
System.out.println("1. 添加考试科目");
System.out.println("2. 添加试题");
System.out.println("3. 查看学生成绩");
System.out.println("4. 查看历史记录");
System.out.println("5. 退出登录");
System.out.print("请选择操作:");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
addSubject();
break;
case 2:
addQuestion();
break;
case 3:
viewStudentScore();
break;
case 4:
viewHistory(null);
break;
case 5:
return;
default:
System.out.println("无效的选择!");
break;
}
}
}
// 参加考试
public void takeExam(Student student) {
System.out.print("请输入科目名称:");
String name = scanner.nextLine();
ExamSubject subject = subjects.get(name);
if (subject == null) {
System.out.println("科目不存在!");
return;
}
ExamRecord record = new ExamRecord(student, subject);
ArrayList<Question> questions = subject.getQuestions();
int i = 1;
for (Question question : questions) {
System.out.println(i + ". " + question);
i++;
}
System.out.println("考试开始!");
long beginTime = System.currentTimeMillis();
while (true) {
long currentTime = System.currentTimeMillis();
if (currentTime - beginTime >= subject.getDuration() * 60 * 1000) {
System.out.println("考试时间到!");
break;
}
System.out.println("剩余时间:" + (subject.getDuration() * 60 - (currentTime - beginTime) / 1000) + "秒");
System.out.print("请输入答案序号(多选题用逗号分隔):");
String answer = scanner.nextLine();
try {
int[] answerIds = parseAnswer(answer);
for (int id : answerIds) {
record.addAnswer(questions.get(i - 1), id);
}
} catch (Exception e) {
System.out.println("答案格式错误!");
}
i++;
if (i > questions.size()) {
System.out.println("考试结束!");
break;
}
}
record.calculateScore();
System.out.println("得分:" + record.getScore());
student.addScore(subject.getName(), record.getScore());
}
// 查看成绩
public void viewScore(Student student) {
System.out.print("请输入科目名称:");
String name = scanner.nextLine();
ExamSubject subject = subjects.get(name);
if (subject == null) {
System.out.println("科目不存在!");
return;
}
Integer score = student.getScores().get(name);
if (score == null) {
System.out.println("还没有参加过该科目的考试!");
} else {
System.out.println("得分:" + score);
}
}
// 查看历史记录
public void viewHistory(User user) {
System.out.print("请输入用户名(留空表示查看所有用户):");
String username = scanner.nextLine();
if ("".equals(username.trim())) {
for (Map.Entry<String, Student> entry : students.entrySet()) {
Student student = entry.getValue();
System.out.println(student.getRealName() + "的历史记录:");
for (ExamRecord record : student.getRecords()) {
System.out.println(record);
}
}
for (Map.Entry<String, Teacher> entry : teachers.entrySet()) {
Teacher teacher = entry.getValue();
System.out.println(teacher.getRealName() + "的历史记录:");
for (ExamRecord record : teacher.getRecords()) {
System.out.println(record);
}
}
} else {
User u = students.get(username);
if (u == null) {
u = teachers.get(username);
if (u == null) {
System.out.println("用户不存在!");
return;
}
}
System.out.println(u.getRealName() + "的历史记录:");
for (ExamRecord record : u.getRecords()) {
System.out.println(record);
}
}
}
// 查看学生成绩
public void viewStudentScore() {
System.out.print("请输入用户名:");
String username = scanner.nextLine();
Student student = students.get(username);
if (student == null) {
System.out.println("学生不存在!");
return;
}
for (Map.Entry<String, Integer> entry : student.getScores().entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
// 解析答案
private int[] parseAnswer(String answer) throws Exception {
String[] split = answer.split(",");
int[] result = new int[split.length];
for (int i = 0; i < split.length; i++) {
try {
result[i] = Integer.parseInt(split[i].trim());
} catch (NumberFormatException e) {
throw new Exception("答案格式错误
相关推荐
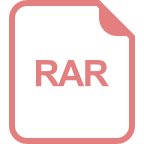
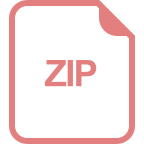
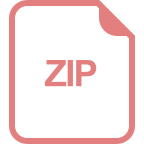














