vue如何实现在网页中预览CAD文件源码
时间: 2024-02-20 11:01:37 浏览: 32
要在网页中预览CAD文件源码,可以使用CAD文件解析库,如Autodesk Forge Viewer或Three.js等。以下是使用Vue.js和Three.js实现在网页中预览CAD文件源码的步骤:
1. 引入Three.js库及CAD文件解析库。
2. 在Vue组件中创建一个canvas元素,用于显示CAD文件。
3. 在Vue组件的mounted钩子函数中,创建Three.js场景、相机、渲染器等对象,并将canvas元素加入到DOM中。
4. 使用CAD文件解析库加载CAD文件,并通过Three.js将其渲染到canvas元素中。
5. 在Vue组件的destroyed钩子函数中,释放资源,如Three.js场景、相机、渲染器等对象。
以下是一个简单的使用Vue.js和Three.js实现在网页中预览CAD文件源码的示例代码:
```html
<template>
<div>
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
import * as THREE from 'three';
import { STLLoader } from 'three/examples/jsm/loaders/STLLoader';
export default {
mounted() {
// 创建Three.js场景、相机、渲染器等对象
this.scene = new THREE.Scene();
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
this.renderer = new THREE.WebGLRenderer({ canvas: this.$refs.canvas });
// 加载CAD文件
const loader = new STLLoader();
loader.load('/path/to/cad/file.stl', geometry => {
// 创建Three.js网格对象并添加到场景中
const material = new THREE.MeshPhongMaterial({ color: 0xff5533, specular: 0x111111, shininess: 200 });
const mesh = new THREE.Mesh(geometry, material);
this.scene.add(mesh);
// 调整相机位置
const center = new THREE.Vector3();
geometry.computeBoundingBox();
geometry.boundingBox.getCenter(center);
mesh.position.sub(center);
this.camera.position.z = Math.max(geometry.boundingBox.max.x, geometry.boundingBox.max.y, geometry.boundingBox.max.z) * 2;
// 开始渲染
this.animate();
});
},
destroyed() {
// 释放资源
this.scene.dispose();
this.camera = null;
this.renderer.dispose();
},
methods: {
animate() {
requestAnimationFrame(this.animate);
this.renderer.render(this.scene, this.camera);
}
}
};
</script>
```
相关推荐
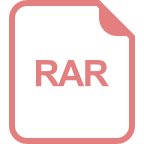














