使用数组记录小明某次考试的各科成绩( 92.0, 89.5, 85.0, 100.0, 90.0, 82.0, 88.0, 93.5, 91.0,78 ) 求小明此次考试的总成绩 求小明此次考试的各科平均分(小数未除尽的情况下截断法保留两位小数)。
时间: 2023-02-12 19:05:41 浏览: 127
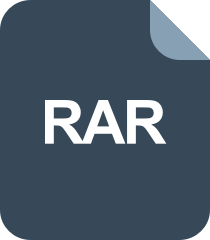
请帮小明同学设计一个程序,输入上次考试成绩(int)和本次考试成绩(int),然后输出成绩提高的百分比,保留两位小数位
使用数组记录小明某次考试的各科成绩,可以这样定义:
```
scores = [92.0, 89.5, 85.0, 100.0, 90.0, 82.0, 88.0, 93.5, 91.0, 78]
```
求小明此次考试的总成绩可以使用 Python 中的内置函数 sum(),代码如下:
```
total_score = sum(scores)
print(total_score)
```
求小明此次考试的各科平均分,可以先求出总分,再除以科目数。代码如下:
```
average_score = round(sum(scores) / len(scores), 2)
print(average_score)
```
其中 round(x,n) 为截断法保留 n 位小数。
阅读全文
相关推荐
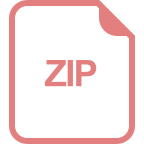
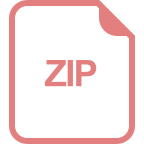
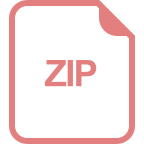
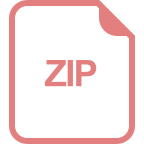
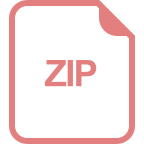
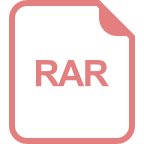
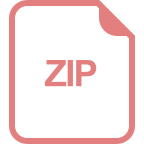
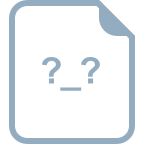
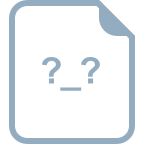
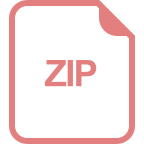
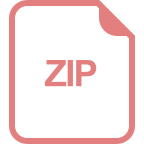
