vue3 xlsx如何将表头加粗
时间: 2023-08-18 18:08:38 浏览: 147
你可以使用 `xlsx-style` 库来设置表头的样式,其中包括加粗等。
首先安装 `xlsx-style`:
```
npm install xlsx-style --save
```
然后在代码中引入:
```javascript
import XLSX from 'xlsx-style'
```
接下来创建一个样式对象:
```javascript
const headerStyle = {
font: {
bold: true
}
}
```
最后在生成 Excel 文件时,将该样式对象传递给表头单元格的 `s` 属性:
```javascript
const worksheet = XLSX.utils.json_to_sheet(data)
worksheet['!cols'] = [{ width: 15 }, { width: 20 }, { width: 25 }]
worksheet['A1'].s = headerStyle
```
以上代码将第一列的宽度设置为 15,第二列的宽度设置为 20,第三列的宽度设置为 25,并将第一行的样式设置为 `headerStyle`。
注意:`xlsx-style` 的使用方法与 `xlsx` 略有不同,详细使用方法可以参考它的文档。
相关问题
vue导出excel文件表头加粗
你可以通过设置样式来使表头加粗。以下是一个示例代码:
```javascript
<template>
<div>
<button @click="exportExcel">导出</button>
</div>
</template>
<script>
import { export_json_to_excel } from '@/utils/excel.js'
export default {
methods: {
exportExcel() {
const header = ['姓名', '年龄', '性别']
const data = [
['张三', 18, '男'],
['李四', 20, '女'],
['王五', 22, '男']
]
const tHeader = header.map(item => {
return { title: item, bold: true }
})
const merges = []
const exportParams = {
header: tHeader,
data,
merges
}
export_json_to_excel(exportParams)
}
}
}
</script>
<style lang="scss">
.bold {
font-weight: bold;
}
</style>
```
注意,在上面的代码中,我们通过设置 `bold` 属性来指定表头是否加粗,然后在样式中设置加粗的样式。在 `excel.js` 文件中,你需要根据 `bold` 的值来设置表头样式。以下是一个示例代码:
```javascript
function generateThead(theme, merges, header) {
const C = []
const CLength = header.length
for (let i = 0; i < CLength; ++i) {
const item = header[i]
const cell = getCell(i, 0)
setCellStyle(cell, theme.cell)
if (item.bold) {
setCellStyle(cell, theme.bold)
}
setText(cell, item.title)
C.push(cell)
}
return [C]
}
```
在上面的代码中,我们首先判断 `item.bold` 的值是否为真,如果是,则设置加粗的样式。你可以根据你自己的需要来设置其他的样式。
vue3导出xlsx并自定义表头
好的,关于vue3导出xlsx并自定义表头的问题,可以采用以下步骤:
1. 安装xlsx插件:npm install xlsx --save
2. 导入插件和自定义表头数据:
```
import XLSX from 'xlsx'
const header = [
{A: '姓名'},
{B: '性别'},
{C: '年龄'}
]
```
3. 将数据格式化为xlsx可以使用的结构:
```
const data = [
{A: '张三', B: '男', C: 18},
{A: '李四', B: '女', C: 20},
{A: '王五', B: '男', C: 22}
]
const sheet = XLSX.utils.json_to_sheet(data, {header: header.map(item => Object.values(item)[0])})
```
4. 生成文件:
```
const wb = XLSX.utils.book_new()
XLSX.utils.book_append_sheet(wb, sheet, 'Sheet1')
XLSX.writeFile(wb, 'test.xlsx')
```
这样就可以导出xlsx文件并自定义表头了。
阅读全文
相关推荐
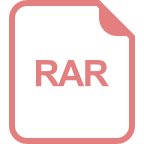
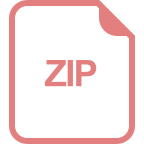
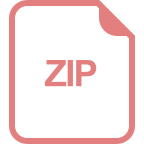












