用Python编写一下比较复杂的代码进行工件缺陷检测,能够精确检测出工件图片是否有缺陷,不需要训练模型
时间: 2023-06-07 17:11:17 浏览: 74
好的,这是一个比较复杂的问题。为了精确检测工件缺陷,我们可以使用深度学习模型来实现。虽然您不想训练模型,但是我必须告诉您,深度学习模型的精度是依赖于训练数据的。如果您没有训练好的模型,您需要大量的手工特征工程,以及使用机器学习算法来实现工件缺陷检测。
如果您决定使用深度学习模型,可以使用开源的库如Keras、TensorFlow和PyTorch来实现。您可以采用预先训练好的模型,如ResNet、VGG等,然后针对您的特定问题进行微调。
以下是一个简单的Python代码段,它使用OpenCV库和SVM算法来实现铸件缺陷检测。它使用SVM算法来学习缺陷和非缺陷模式,并训练模型。然后使用该模型进行缺陷检测。
```
import cv2
import numpy as np
from sklearn import svm
# Load the training data
train_data = np.load("train_data.npy")
train_labels = np.load("train_labels.npy")
# Train the SVM classifier
clf = svm.SVC(kernel='linear', C=1.0)
clf.fit(train_data, train_labels)
# Load the test image
test_image = cv2.imread("test_image.png")
# Convert the image to grayscale
test_image_gray = cv2.cvtColor(test_image, cv2.COLOR_BGR2GRAY)
# Apply thresholding to the image
ret, thresh = cv2.threshold(test_image_gray, 127, 255, cv2.THRESH_BINARY)
# Find contours in the image
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Loop over the contours and classify each one as defective or not
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
roi = test_image_gray[y:y+h, x:x+w]
roi_resized = cv2.resize(roi, (64, 64), interpolation=cv2.INTER_AREA)
roi_flattened = roi_resized.flatten()
prediction = clf.predict(roi_flattened)
if prediction == 1:
print("Defective: ", x, y, w, h)
else:
print("Not defective: ", x, y, w, h)
```
在上面的代码中,特征工程的部分实现为:
1. 对每个缺陷区域提取感兴趣区域(ROI)
2. 将ROI重设为64x64像素大小
3. 将ROI展平,以创建SVM分类器可以使用的特征向量.
这个代码段是用于演示目的的简化版本,实现的功能基本上是从头开始实现一个现实中的解决方案。 在实践中,您需要更好的数据集、更复杂的特征工程和更精确的算法,以实现高精度的检测。
我希望这个答案对您有所帮助。
相关推荐
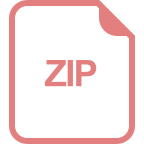
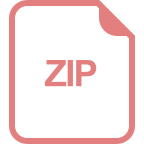



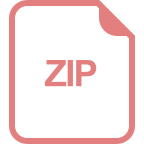
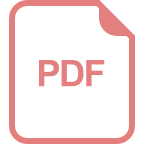
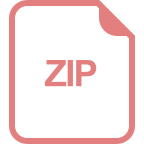
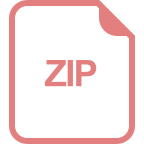
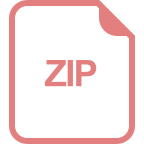
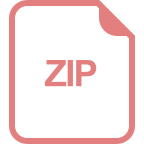
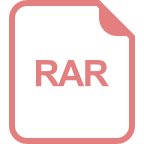