binance websocket获取深度5档100ms
时间: 2023-06-13 17:03:42 浏览: 49
以下是使用Python的Binance API和Websocket获取深度5档100ms的示例代码:
```python
from binance.client import Client
from binance.websockets import BinanceSocketManager
api_key = 'your_api_key'
api_secret = 'your_api_secret'
client = Client(api_key, api_secret)
def process_message(msg):
print(msg)
bm = BinanceSocketManager(client)
conn_key = bm.start_depth_socket('BTCUSDT', process_message, depth=5, interval=100)
bm.start()
```
这段代码使用Binance API和Websocket创建了一个深度5档100ms的实时数据流,每当有新数据时,将触发`process_message`函数打印出来。你可以将`'BTCUSDT'`替换为你所需的货币对。
相关问题
binance websocket获取深度100ms
要使用Binance WebSocket API获取深度数据,您需要按照以下步骤进行操作:
1. 连接到Binance WebSocket服务器
您可以使用以下URL连接到Binance WebSocket服务器:
wss://stream.binance.com:9443/ws
2. 订阅深度数据
使用以下格式订阅深度数据:
{
"method": "SUBSCRIBE",
"params":
[
"btcusdt@depth@100ms"
],
"id": 1
}
其中,btcusdt是您要订阅深度数据的交易对,100ms是更新深度数据的频率。
3. 处理深度数据
一旦您成功订阅深度数据,您将收到每100毫秒更新一次的深度数据。您可以使用以下格式处理深度数据:
{
"e": "depthUpdate",
"E": 123456789, // 事件时间
"s": "BTCUSDT", // 交易对
"U": 456, // 第一条更新的ID
"u": 789, // 最后一条更新的ID
"b": // 买单列表
[
[
"0.0024", // 价格
"10" // 数量
]
],
"a": // 卖单列表
[
[
"0.0025", // 价格
"20" // 数量
]
]
}
其中,b表示买单列表,a表示卖单列表。每个列表都包含一组价格和数量。
请注意,您需要在连接到Binance WebSocket服务器后,立即订阅深度数据。否则,您可能会错过深度数据的更新。
binance websocket获取深度
要使用Binance Websocket API获取深度信息,您需要使用以下步骤:
1. 订阅深度信息
2. 接收深度信息
3. 处理深度信息
首先,您需要使用以下代码订阅深度信息:
```python
import websocket
import json
symbol = "btcusdt"
depth = "5"
def on_open(ws):
params = {
"method": "SUBSCRIBE",
"params":
[
f"{symbol}@depth{depth}"
],
"id": 1
}
ws.send(json.dumps(params))
def on_message(ws, message):
message = json.loads(message)
if message['e'] == 'depthUpdate':
print(message)
ws = websocket.WebSocketApp("wss://stream.binance.com:9443/ws",
on_open = on_open,
on_message = on_message)
ws.run_forever()
```
在上面的代码中,我们使用websocket库连接到Binance的websocket API,并在on_open回调函数中向API发送订阅请求。我们使用了btcusdt作为交易对,depth为5,表示我们只需要5档深度信息。在on_message回调函数中,我们处理收到的深度信息。
请注意:在Binance的Websocket API中,每个连接只能订阅一个交易对的深度信息。如果您需要订阅多个交易对,您需要建立多个连接。
接下来,我们将处理深度信息。深度信息是一个JSON对象,它包含了最新的买卖盘信息。在我们的on_message回调函数中,我们可以使用以下代码处理深度信息:
```python
def on_message(ws, message):
message = json.loads(message)
if message['e'] == 'depthUpdate':
bids = message['b']
asks = message['a']
for bid in bids:
price = float(bid[0])
quantity = float(bid[1])
print(f"Bid Price: {price}, Quantity: {quantity}")
for ask in asks:
price = float(ask[0])
quantity = float(ask[1])
print(f"Ask Price: {price}, Quantity: {quantity}")
```
在上面的代码中,我们首先获取最新的买盘信息和卖盘信息,并使用for循环遍历它们。对于每个买单和卖单,我们获取价格和数量,并将它们打印出来。
这样,您就可以使用Binance Websocket API获取深度信息了。
相关推荐
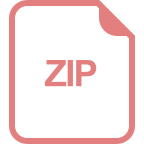
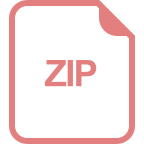
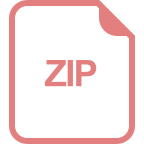












