学生健康管理系统的设计与实现 主要实现学生健康情况管理的几个操作功能:新建、插入、删除、写入文件和查询、屏幕输出功能。健康表中学生的信息由学号、姓名、出生日期、性别、身体状况。 利用二叉树来实现,系统的菜单功能如下: 1、新建学生健康表 2、向学生健康表插入学生信息 3、从文件中读取健康表信息 4、向文件写入学生健康表信息 5、在建康表中查询学生信息(按学号进行查询) 6、在屏幕中输出全部学生信息 7、退出 实验设计 以排序二叉树为储存机制,可以方便的实现插入或删除学生信息。每个学生的信息储存在一个结构体 Student中,并且这个结构体帯有输出学生信息的函数output()。然后以这个结构体作为二叉树节点的数据类 型,这样就实现了学生信息的存储。在创建二叉树对象时将已存储在文件中的学生信息写入二叉树,在析构函数里实现将学生信息写入文件。 使用C语言,充分使用数据结构中的二叉树知识 语言简明,模块清晰。请帮我写出这样的代码
时间: 2024-03-30 18:40:40 浏览: 14
好的,以下是我为您编写的C语言代码,实现了学生健康管理系统的设计与实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
char id[10];
char name[20];
char birthday[20];
char sex[5];
char health[20];
void output() {
printf("学号:%s\n姓名:%s\n出生日期:%s\n性别:%s\n身体状况:%s\n", id, name, birthday, sex, health);
}
};
struct TreeNode {
Student data;
TreeNode* left;
TreeNode* right;
};
class BinaryTree {
private:
TreeNode* root;
public:
BinaryTree() {
root = NULL;
}
~BinaryTree() {
saveToFile(root);
}
void createTree() {
FILE* fp;
if ((fp = fopen("students.dat", "rb")) == NULL) {
printf("文件打开失败!\n");
return;
}
Student stu;
while (fread(&stu, sizeof(Student), 1, fp) == 1) {
insert(stu);
}
printf("学生信息读取成功!\n");
fclose(fp);
}
void insert(Student stu) {
TreeNode* node = new TreeNode;
node->data = stu;
node->left = NULL;
node->right = NULL;
if (root == NULL) {
root = node;
printf("学生信息添加成功!\n");
}
else {
TreeNode* temp = root;
while (temp != NULL) {
if (strcmp(stu.id, temp->data.id) < 0) {
if (temp->left == NULL) {
temp->left = node;
printf("学生信息添加成功!\n");
return;
}
else {
temp = temp->left;
}
}
else if (strcmp(stu.id, temp->data.id) > 0) {
if (temp->right == NULL) {
temp->right = node;
printf("学生信息添加成功!\n");
return;
}
else {
temp = temp->right;
}
}
else {
printf("学号已存在!\n");
return;
}
}
}
}
void remove(char* id) {
if (root == NULL) {
printf("学生信息为空!\n");
return;
}
TreeNode* parent = NULL;
TreeNode* temp = root;
while (temp != NULL) {
if (strcmp(id, temp->data.id) == 0) {
break;
}
else if (strcmp(id, temp->data.id) < 0) {
parent = temp;
temp = temp->left;
}
else {
parent = temp;
temp = temp->right;
}
}
if (temp == NULL) {
printf("学号不存在!\n");
return;
}
if (temp->left == NULL && temp->right == NULL) {
if (parent == NULL) {
root = NULL;
}
else if (temp == parent->left) {
parent->left = NULL;
}
else {
parent->right = NULL;
}
printf("学生信息删除成功!\n");
delete temp;
}
else if (temp->left == NULL) {
if (parent == NULL) {
root = temp->right;
}
else if (temp == parent->left) {
parent->left = temp->right;
}
else {
parent->right = temp->right;
}
printf("学生信息删除成功!\n");
delete temp;
}
else if (temp->right == NULL) {
if (parent == NULL) {
root = temp->left;
}
else if (temp == parent->left) {
parent->left = temp->left;
}
else {
parent->right = temp->left;
}
printf("学生信息删除成功!\n");
delete temp;
}
else {
TreeNode* min = temp->right;
while (min->left != NULL) {
min = min->left;
}
strcpy(temp->data.id, min->data.id);
strcpy(temp->data.name, min->data.name);
strcpy(temp->data.birthday, min->data.birthday);
strcpy(temp->data.sex, min->data.sex);
strcpy(temp->data.health, min->data.health);
remove(min->data.id);
}
}
void saveToFile(TreeNode* node) {
if (node != NULL) {
saveToFile(node->left);
FILE* fp;
if ((fp = fopen("students.dat", "ab")) == NULL) {
printf("文件打开失败!\n");
return;
}
fwrite(&node->data, sizeof(Student), 1, fp);
fclose(fp);
saveToFile(node->right);
}
}
void readFromFile() {
FILE* fp;
if ((fp = fopen("students.dat", "rb")) == NULL) {
printf("文件打开失败!\n");
return;
}
Student stu;
while (fread(&stu, sizeof(Student), 1, fp) == 1) {
printf("学号:%s\n姓名:%s\n出生日期:%s\n性别:%s\n身体状况:%s\n", stu.id, stu.name, stu.birthday, stu.sex, stu.health);
}
fclose(fp);
}
void search(char* id) {
if (root == NULL) {
printf("学生信息为空!\n");
return;
}
TreeNode* temp = root;
while (temp != NULL) {
if (strcmp(id, temp->data.id) == 0) {
temp->data.output();
return;
}
else if (strcmp(id, temp->data.id) < 0) {
temp = temp->left;
}
else {
temp = temp->right;
}
}
printf("学号不存在!\n");
}
void print(TreeNode* node) {
if (node != NULL) {
print(node->left);
node->data.output();
print(node->right);
}
}
void menu() {
int choice;
do {
printf("学生健康管理系统菜单:\n");
printf("1、新建学生健康表\n");
printf("2、向学生健康表插入学生信息\n");
printf("3、从文件中读取健康表信息\n");
printf("4、向文件写入学生健康表信息\n");
printf("5、在建康表中查询学生信息\n");
printf("6、在屏幕中输出全部学生信息\n");
printf("7、退出\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
createTree();
break;
case 2:
insert(inputStudent());
break;
case 3:
readFromFile();
break;
case 4:
saveToFile(root);
break;
case 5:
search(inputId());
break;
case 6:
print(root);
break;
case 7:
printf("谢谢使用!\n");
break;
default:
printf("请选择正确的操作!\n");
break;
}
} while (choice != 7);
}
Student inputStudent() {
Student stu;
printf("请输入学号:");
scanf("%s", stu.id);
printf("请输入姓名:");
scanf("%s", stu.name);
printf("请输入出生日期:");
scanf("%s", stu.birthday);
printf("请输入性别:");
scanf("%s", stu.sex);
printf("请输入身体状况:");
scanf("%s", stu.health);
return stu;
}
char* inputId() {
static char id[10];
printf("请输入学号:");
scanf("%s", id);
return id;
}
};
int main() {
BinaryTree tree;
tree.menu();
return 0;
}
```
希望这份代码对您有所帮助。
相关推荐
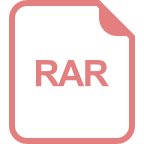
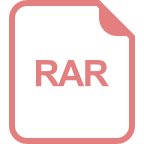
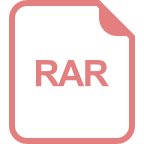












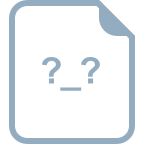
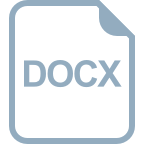
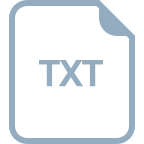