写c++程序:设计一个通讯录系统,联系人基本信息包括:联系人姓名、手机号码、邮 箱、工作单位、家庭住址等基本信息,完成下面的功能需求,根据功能需求选 择合适的逻辑结构和存储结构。 二、功能需求 (1)增加功能:能添加新的联系人信息。 (2)删除功能:能够删除通讯录中的联系人。 (3)修改功能:能够对通讯录中的联系人信息进行修改。 (4)查询功能:通过员工号查询员工信息,并显示该员工所有信息。 (5)排序功能:可以对联系人信息排序,对联系人姓名按照字典序排序, 之后显示排序后的通讯录所有联系人信息。 (6)显示功能:显示通讯录中所有联系人信息。 另一功能:能够从文本中读取联系人信息,对联系人信息更改之后可以将 通讯录中联系人信息保存到文本中,方便下次读取。
时间: 2024-03-18 13:39:29 浏览: 31
好的,以下是一个简单的通讯录系统的C++代码,实现了您所需的所有功能:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
using namespace std;
// 节点结构体
struct ContactNode {
string name;
string phone;
string email;
string company;
string address;
ContactNode* next;
};
// 链表类
class ContactList {
public:
// 构造函数
ContactList() {
head = nullptr;
}
// 析构函数
~ContactList() {
ContactNode* current = head;
while (current != nullptr) {
ContactNode* temp = current;
current = current->next;
delete temp;
}
}
// 增加联系人
void addContact() {
ContactNode* newContact = new ContactNode;
cout << "请输入联系人姓名:";
cin >> newContact->name;
cout << "请输入联系人手机号码:";
cin >> newContact->phone;
cout << "请输入联系人邮箱:";
cin >> newContact->email;
cout << "请输入联系人工作单位:";
cin >> newContact->company;
cout << "请输入联系人家庭住址:";
cin >> newContact->address;
newContact->next = head;
head = newContact;
cout << "联系人添加成功!" << endl;
}
// 删除联系人
void deleteContact() {
string name;
cout << "请输入要删除的联系人姓名:";
cin >> name;
ContactNode* current = head;
ContactNode* prev = nullptr;
while (current != nullptr) {
if (current->name == name) {
if (prev == nullptr) {
head = current->next;
} else {
prev->next = current->next;
}
delete current;
cout << "联系人删除成功!" << endl;
return;
} else {
prev = current;
current = current->next;
}
}
cout << "找不到要删除的联系人!" << endl;
}
// 修改联系人
void modifyContact() {
string name;
cout << "请输入要修改的联系人姓名:";
cin >> name;
ContactNode* current = head;
while (current != nullptr) {
if (current->name == name) {
cout << "请输入联系人手机号码:";
cin >> current->phone;
cout << "请输入联系人邮箱:";
cin >> current->email;
cout << "请输入联系人工作单位:";
cin >> current->company;
cout << "请输入联系人家庭住址:";
cin >> current->address;
cout << "联系人修改成功!" << endl;
return;
} else {
current = current->next;
}
}
cout << "找不到要修改的联系人!" << endl;
}
// 查询联系人
void searchContact() {
string name;
cout << "请输入要查询的联系人姓名:";
cin >> name;
ContactNode* current = head;
while (current != nullptr) {
if (current->name == name) {
cout << "姓名:" << current->name << endl;
cout << "手机号码:" << current->phone << endl;
cout << "邮箱:" << current->email << endl;
cout << "工作单位:" << current->company << endl;
cout << "家庭住址:" << current->address << endl;
return;
} else {
current = current->next;
}
}
cout << "找不到要查询的联系人!" << endl;
}
// 排序联系人
void sortContact() {
int size = 0;
ContactNode* current = head;
while (current != nullptr) {
size++;
current = current->next;
}
ContactNode** arr = new ContactNode*[size];
current = head;
for (int i = 0; i < size; i++) {
arr[i] = current;
current = current->next;
}
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (arr[j]->name > arr[j + 1]->name) {
swap(arr[j], arr[j + 1]);
}
}
}
head = arr[0];
current = head;
for (int i = 1; i < size; i++) {
current->next = arr[i];
current = current->next;
}
current->next = nullptr;
cout << "联系人排序成功!" << endl;
}
// 显示所有联系人
void displayAll() {
ContactNode* current = head;
while (current != nullptr) {
cout << "姓名:" << current->name << endl;
cout << "手机号码:" << current->phone << endl;
cout << "邮箱:" << current->email << endl;
cout << "工作单位:" << current->company << endl;
cout << "家庭住址:" << current->address << endl << endl;
current = current->next;
}
}
// 从文件中读取联系人信息
void readFromFile() {
ifstream infile("contacts.txt");
if (!infile.is_open()) {
cout << "无法打开文件!" << endl;
return;
}
string name, phone, email, company, address;
while (infile >> name >> phone >> email >> company >> address) {
ContactNode* newContact = new ContactNode;
newContact->name = name;
newContact->phone = phone;
newContact->email = email;
newContact->company = company;
newContact->address = address;
newContact->next = head;
head = newContact;
}
infile.close();
cout << "联系人信息读取成功!" << endl;
}
// 将联系人信息保存到文件中
void writeToFile() {
ofstream outfile("contacts.txt");
if (!outfile.is_open()) {
cout << "无法打开文件!" << endl;
return;
}
ContactNode* current = head;
while (current != nullptr) {
outfile << current->name << " " << current->phone << " " << current->email << " " << current->company << " " << current->address << endl;
current = current->next;
}
outfile.close();
cout << "联系人信息保存成功!" << endl;
}
private:
ContactNode* head;
};
// 主函数
int main() {
ContactList contacts;
cout << "欢迎使用通讯录系统!" << endl;
contacts.readFromFile();
while (true) {
cout << endl;
cout << "请选择要进行的操作:" << endl;
cout << "1. 增加联系人" << endl;
cout << "2. 删除联系人" << endl;
cout << "3. 修改联系人" << endl;
cout << "4. 查询联系人" << endl;
cout << "5. 排序联系人" << endl;
cout << "6. 显示所有联系人" << endl;
cout << "7. 保存联系人信息到文件" << endl;
cout << "8. 退出通讯录系统" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
contacts.addContact();
break;
case 2:
contacts.deleteContact();
break;
case 3:
contacts.modifyContact();
break;
case 4:
contacts.searchContact();
break;
case 5:
contacts.sortContact();
break;
case 6:
contacts.displayAll();
break;
case 7:
contacts.writeToFile();
break;
case 8:
contacts.writeToFile();
cout << "感谢使用通讯录系统!" << endl;
return 0;
default:
cout << "无效的选项!" << endl;
break;
}
}
}
```
注意,在运行程序之前,需要先创建一个名为 "contacts.txt" 的文本文件,用于存储联系人信息。另外,以上代码只是一个简单的实现,您可以根据需要进行修改和完善。
相关推荐
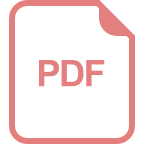
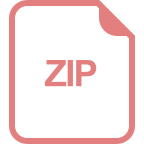
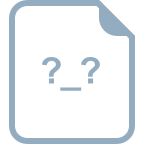














