用原子操作比较并交换实现对共享内存的读写锁机制,用C语言编程并详细解析
时间: 2024-04-29 13:21:23 浏览: 12
读写锁是一种用于多线程编程中的同步机制,它可以提高多线程程序的并发性能。读写锁允许多个线程同时读取共享资源,但是当有一个线程写入共享资源时,其他读取和写入操作都必须等待。在本篇文章中,我们将使用原子操作比较并交换实现对共享内存的读写锁机制。
首先,我们需要定义一个共享内存区域和两个计数器:一个用于记录当前正在读取共享内存的线程数量,另一个用于记录当前正在写入共享内存的线程数量。这些变量应该在多个线程之间共享。
```c
#include <stdatomic.h>
#define MAX_THREADS 100
typedef struct {
atomic_int read_count;
atomic_int write_count;
char shared_data[1024];
} shared_memory_t;
shared_memory_t shared_memory;
```
接下来,我们需要实现读取和写入锁。这些锁可以使用原子操作比较并交换实现。对于读取锁,我们需要使用原子操作递增读取计数器。如果当前没有线程正在写入共享内存,那么读取操作可以继续执行。否则,读取线程必须等待所有写入线程完成,然后才能开始读取共享内存。对于写入锁,我们需要使用原子操作设置写入计数器为1。如果当前没有线程正在读取或写入共享内存,那么写入操作可以继续执行。否则,写入线程必须等待所有读取和写入线程完成,然后才能开始写入共享内存。
```c
void read_lock() {
while (1) {
int write_count = atomic_load(&shared_memory.write_count);
if (write_count == 0) {
atomic_fetch_add(&shared_memory.read_count, 1);
break;
}
}
}
void read_unlock() {
atomic_fetch_sub(&shared_memory.read_count, 1);
}
void write_lock() {
while (1) {
int write_count = atomic_load(&shared_memory.write_count);
int read_count = atomic_load(&shared_memory.read_count);
if (write_count == 0 && read_count == 0 && atomic_compare_exchange_weak(&shared_memory.write_count, &write_count, 1)) {
break;
}
}
}
void write_unlock() {
atomic_fetch_sub(&shared_memory.write_count, 1);
}
```
最后,我们可以使用这些锁来保护共享内存。例如,下面的代码展示了如何在多个线程之间读取和写入共享内存。
```c
void* reader(void* arg) {
read_lock();
printf("Read: %s\n", shared_memory.shared_data);
read_unlock();
}
void* writer(void* arg) {
write_lock();
sprintf(shared_memory.shared_data, "Hello, world!");
write_unlock();
}
int main() {
pthread_t threads[MAX_THREADS];
int i;
for (i = 0; i < 10; i++) {
pthread_create(&threads[i], NULL, reader, NULL);
}
for (i = 10; i < 20; i++) {
pthread_create(&threads[i], NULL, writer, NULL);
}
for (i = 0; i < 20; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
```
在这个例子中,我们创建了10个读取线程和10个写入线程,并且使用读取锁和写入锁来保护共享内存。这样,我们就实现了对共享内存的读写锁机制。
相关推荐
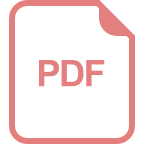
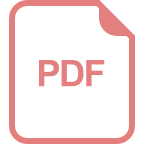
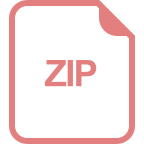














